Before we starts with JSON Schema validation, let’s first explore what is JSON,
What is JSON?
JSON stands for JavaScript Object Notation. It is a lightweight format for storing and transporting data and often used when data is sent from server to webpages. It is self describing and easy to understand.
The following are important syntax rules for JSON:
Data is in name/value pairs.
Data is separated by commas.
Curly braces hold objects.
Square brackets hold arrays.
{
"page": 1,
"totalpages": 2,
"employeedata":
[
{
"id": 1,
"firstname": "Michael",
"lastname": "Doe",
"designation": "QA",
"location": "Remote"
},
{
"id": 2,
"firstname": "Johnny",
"lastname": "Ford",
"designation": "QA",
"location": "NY,US"
}
],
"company": {
"name": "QA Inc",
"Address": "New Jersey, US"
}
}
JSON Validation - Syntactic vs Semantic
When we validate a JSON document without its Schema, we are validating only the syntax of the document. It can be done as
Syntactic validation guarantees only that the document is well-formed.The tools such as JSONLint, and the JSON parsers perform only Syntactic validations.
Semantic validation is more than syntactic validation. It performs the syntax checks as well as the data checks.It helps to ensure that the client is sending only allowed fields in JSON documents and not any invalid name-value pairs. It can also help in checking the format of a phone number, a date/time, a postal code, an email address, or a credit card number.
What Is JSON Schema?
JSON Schema is a specification for JSON-based format that defines the structure, format, and constraints of JSON documents/data. As JSON Schema provides complete structural validation, it helps in automated tests and also validating the client-submitted data for verification. It provides a way to describe the expected structure of JSON data, making it a valuable tool for ensuring the integrity of data exchanged between API clients and servers.
JSON Schema Structure
JSON Schema Version: The JSON Schema document should begin by specifying the version of the JSON Schema specification it adheres to. This is done using the "$schema" keyword, which indicates the URI of the schema version being used.
For example:
{
"$schema": "http://json-schema.org/draft-07/schema#",
// Link varies depending on its version
// Rest of the schema definition
}
Type Definitions: The core of a JSON Schema defines the data types and constraints for the JSON document. The most common data types include:
"object": Represents a JSON object (a collection of key-value pairs).
"array": Represents a JSON array (an ordered list of values).
"string": Represents a JSON string (a collection of characters).
"number": Represents a JSON number (integer or floating-point).
"boolean": Represents a JSON boolean (true or false).
"null": Represents a JSON null value.
For example:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object", // Rest of the schema definition
}
Properties and Items: For objects and arrays, you can define their properties and items. Properties describe the expected key-value pairs in an object, while items describe the expected elements in an array. You can use the "properties" and "items" keywords to define these, respectively:
For objects:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties":
{
"name": {"type": "string"},
"age": {"type": "integer"}
}
}
For arrays:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "array",
"items":
{
"type": "string"
}
}
Required Properties: You can specify which properties within an object are required using the "required" keyword:
{
"$schema": "http://json-schema.org/draft-07/schema#",
"type": "object",
"properties":
{
"name": { "type": "string" },
"age": { "type": "integer" }
}, "required": ["name"]
}
Additional Constraints: JSON Schemas allow you to specify additional constraints, such as minimum and maximum values for numbers, regular expressions for strings, and more, depending on the data type. These constraints are specified using various keywords specific to the data type.
How To Generate JSON Schema
You can write a JSON schema in REST assured in two ways:
Manually - you write the whole set of code by yourself.
Using the JSON schema tool - you provide the JSON object as input. This tool returns a JSON schema in REST assured.
Here im going with JSON schema tool available online with the following link
For example:
If you have the following JSON data
{
"order_id": "1234567890",
"order_description": "sample description",
"order_status": "Processed",
"last_updated_timestamp": "1671916800000",
"special_order": false
}
And with the help of tool by using above link,we can generate the JSON Schema just by copy pasting the JSON data on to the document field (like below) and clicking on Generate Schema button

Now you will get the JSON Schema as below
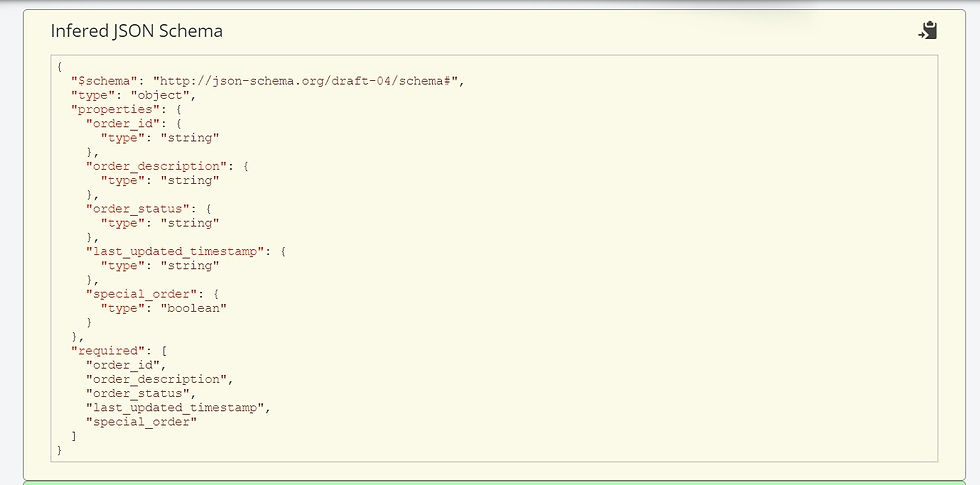
Now let’s implement it all into a rest assured test:
@Test
public void testJsonSchema()
{
String baseUri = "https://my-json-server.typicode.com";
given().baseUri(baseUri)
.when().get("/darmawanh/processOrder/success")
.then().assertThat().statusCode(200)
.body(JsonSchemaValidator.matchesJsonSchemaInClasspath("schemavalidation.json"))
.extract().response();
}
as below in eclipse:
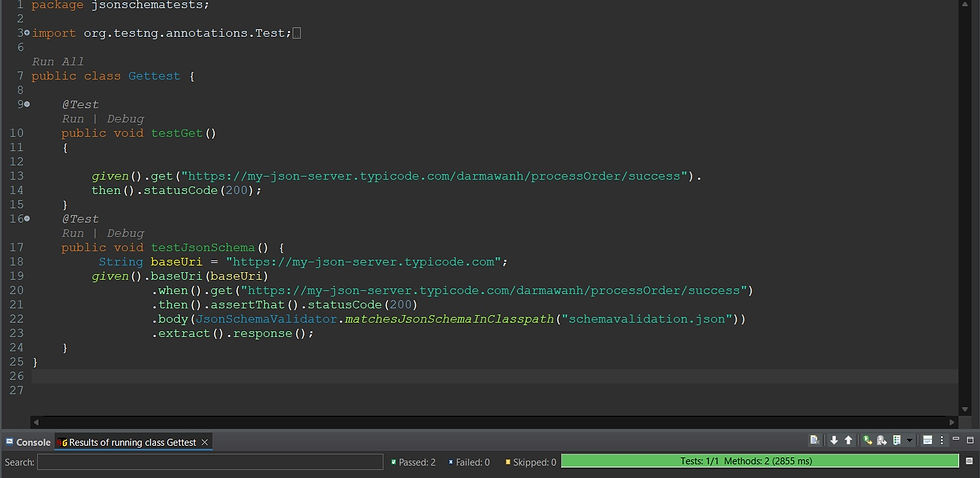
you will get the result as follows:
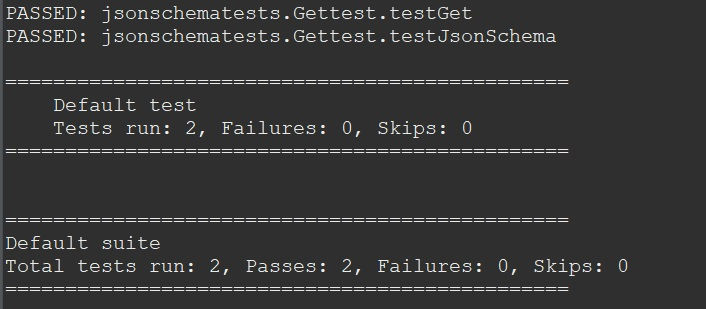
Code explanation:
In the above example, we’re using JsonSchemaValidator.matchesJsonSchemaInClasspath to validate the API response against a JSON Schema located in your classpath. Make sure to replace "yourjsonschema.json" with the actual path to your JSON Schema file.
Thanks!!
Keep Learning!!