In this blog you can find information on What , Why and How to use the following in Selenium Project .
Topics Covered
Framework
PageObjects
PageFactory
Pom.xml
Maven Dependencies

What is a Selenium framework?
Selenium framework is a code structure for making code maintenance simpler, and code readability better. A framework involves breaking the entire code into smaller pieces of code, which test a particular functionality.
The code is structured such that, the “data set” is separated from the actual “test case” which will test the functionality of the web application.
Benefits of Selenium framework
Increased code reusage
Improved code readability
Higher portability
Reduced script maintenance
Customized /User Defined Framework
*Modular Driven Framework -
Modular framework is like creation of small, independent scripts that represents modules, sections and functions of the application under test. Testers use Modular testing framework to divide an application into multiple modules and create test scripts individually.
*Data- driven Framework - Taking data from Excel
A Data Driven Framework in Selenium is a technique of separating the “data set” from the actual “test case” (code). Since the test case is separated from the data set, one can easily modify the test case of a particular functionality without making changes to the code.
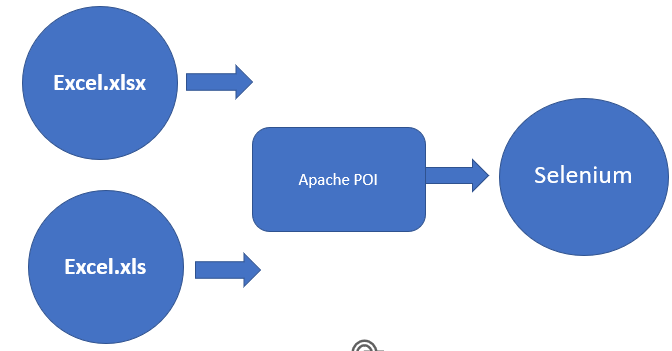
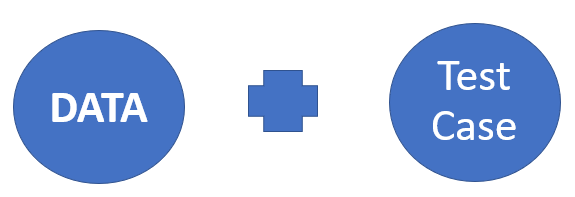
*Keyword driven Framework - Call functions by Keywords
A keyword driven framework in Selenium is a testing approach that uses a table of keywords to represent the actions and inputs for each test case. The test cases are then constructed by calling the keywords in a specific sequence to perform the desired testing steps.

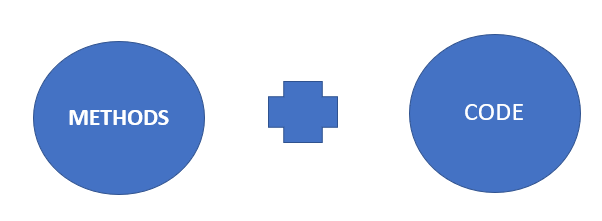
*Hybrid Framework -
Hybrid Driven Framework in Selenium is a mix of both the Data-Driven and Keyword Driven frameworks. In this case, the keywords as well as the test data, are externalized. Keywords are stored in a separate Java class file and test data can be maintained in a Properties file or an Excel file.
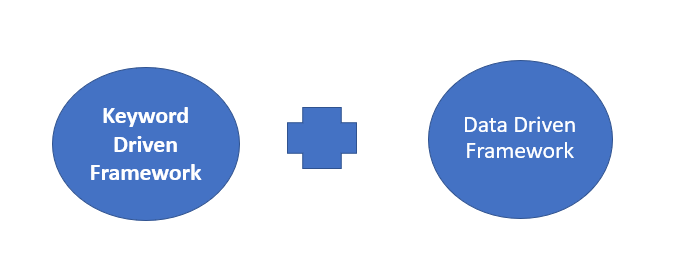
What is the difference between Page Object Model (POM) and Page Factory:
Page Object
Is a class that represents a web page and hold the functionality and members.
Page Object Model, also known as POM, is a design pattern in Selenium that creates an object repository for storing all web elements.
It helps reduce code duplication and improves test case maintenance.
In Page Object Model, consider each web page of an application as a class file.
Page Factory
Is a way to initialize the web elements you want to interact with within the page object when you create an instance of it.
Page Factory is a class provided by Selenium WebDriver to support Page Object Design patterns.
In Page Factory, testers use @FindBy annotation.
The initElements method is used to initialize web elements.
@FindBy: An annotation used in Page Factory to locate and declare web elements using different locators.
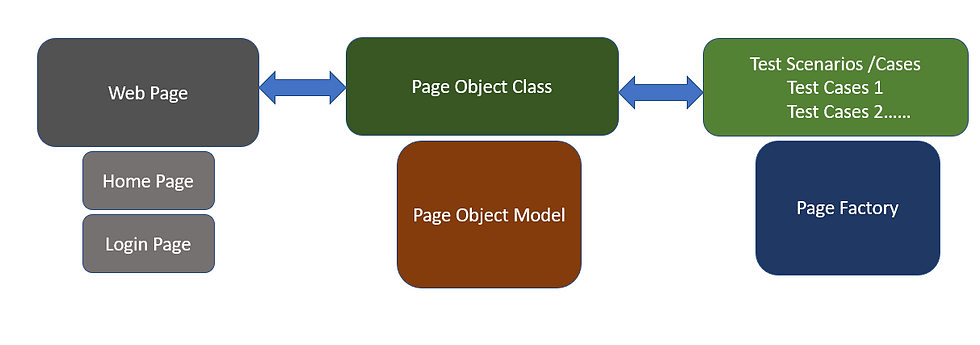
What is the use of POM?
Object repository is independent of test cases.
Code becomes less and optimized because of the reusable page methods in the POM classes.
Methods get more realistic names which can be easily mapped with the
operation happening in UI.
1.Using FindBy
import org.openqa.selenium.support.FindBy;
import org.openqa.selenium.support.How;
public class Login_pageObjects
{
@FindBy(how=How.XPATH, using="//input[@id='Email']")
public static WebElement username;
@FindBy(how=How.XPATH,using="//input[@id='Password']")
public static WebElement password;
@FindBy(how=How.XPATH,using="//button[normalize-space()='Log in']")
public static WebElement loginButton;
}
2.Using By
import org.openqa.selenium.By;
public class Login_pageObjects
{
By username=By.name("username");
By password=By.name("password");
By loginButton=By.xpath("//button[@type='submit']");
}
Sample Code for Orange HRM Using POM
public class OrangeHRM_POM {
WebDriver driver;
String URL="https://opensource-demo.orangehrmlive.com/web/index.php/auth/login";
// 1.*************************************
By userName=By.name("username");
By passWord=By.name("password");
By logIn=By.xpath("//button[@type='submit']");
// 2.*******************************************
By info=By.xpath("//*[@id='app']//ul/li[6]/a");
By addContact=By.linkText("Contact Details");
By street1=By.xpath("//*[@id='app']//form/div[1]/div/div[1]/div/div[2]/input");
By street2= By.xpath("//*[@id='app']//form/div[1]/div/div[2]/div/div[2]/input");
By city=By.xpath("//*[@id='app']//form/div[1]/div/div[3]/div/div[2]/input");
By state=By.xpath("//*[@id='app']//form/div[1]/div/div[4]/div/div[2]/input");
By zip=By.xpath("//*[@id='app']//form/div[1]/div/div[5]/div/div[2]/input");
By telephone=By.xpath("//*[@id='app']//form/div[2]/div/div[1]/div/div[2]/input");
By saveInfo=By.xpath("//*[@id='app']//form/div[4]/button");
// launching browser
@Test(priority=1)
public void launch_Browser() {
//1.Launch the application http://tutorialsninja.com/demo/index.php
System.setProperty("webdriver.chrome.driver","C:\\Users\\Reka\\Drivers\\chromedriver.exe");
driver=new ChromeDriver();
}
// 1. Launch the below application https://opensource-demo.orangehrmlive.com/web/index.php/auth/login
//and login with give credentials
@Test(priority=2)
public void loginPage() {
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
driver.get(URL);
driver.findElement(userName).sendKeys("Admin");
driver.findElement(passWord).sendKeys("admin123");
driver.findElement(logIn).click();
}
//2. Navigate to My info - > Contact Details, enter contact details and press save button
@Test(priority=3)
public void myInfo() throws InterruptedException {
driver.findElement(info).click();
driver.findElement(addContact).click();
//driver.findElement(street1).clear();
driver.findElement(street1).sendKeys(Keys.CONTROL + "A");
driver.findElement(street1).sendKeys(Keys.CONTROL + "X");
driver.findElement(street1).sendKeys("independence");
driver.findElement(street2).sendKeys(Keys.CONTROL + "A");
driver.findElement(street2).sendKeys(Keys.CONTROL + "X");
driver.findElement(street2).sendKeys("coit");
//driver.findElement(city).clear();
driver.findElement(city).sendKeys(Keys.CONTROL + "A");
driver.findElement(city).sendKeys(Keys.CONTROL + "X");
driver.findElement(city).sendKeys("chennai");
//driver.findElement(state).clear();
driver.findElement(state).sendKeys(Keys.CONTROL + "A");
driver.findElement(state).sendKeys(Keys.CONTROL + "X");
driver.findElement(state).sendKeys("TN");
driver.findElement(zip).sendKeys(Keys.CONTROL + "A");
driver.findElement(zip).sendKeys(Keys.CONTROL + "X");
//driver.findElement(zip).clear();
driver.findElement(zip).sendKeys("60000");
//driver.findElement(telephone).clear();
driver.findElement(telephone).sendKeys(Keys.CONTROL + "A");
driver.findElement(telephone).sendKeys(Keys.CONTROL + "X");
driver.findElement(telephone).sendKeys("1234567890");
driver.findElement(saveInfo).click() ;
}
}
Difference between POM and PF
In POM (PAGE OBJECT MODEL)
Webelement Locators
By userName=By.name("username");
By passWord=By.name("password");
Using Elements In Method
driver.findElement(userName).sendKeys("Admin");
driver.findElement(passWord).sendKeys("admin123");
In PF (PAGE FACTORY)
Webelement Locators
@FindBy(id="email")
public static WebElement e_Mail;
@FindBy(id="password")
publicstatic WebElement pass_Word;
Using page Factory to use all webelements
PageFactory.initElements(driver, Juice_Shop_PF.class); // for null exception
Using Elements in method
e_Mail.sendKeys("admin069@gmail.com");
pass_Word.sendKeys("admin1234");
PAGE FACTORY Example
public void login() throws InterruptedException
{
WebDriverManager.chromedriver().setup();
WebDriver driver=new ChromeDriver();
driver.get("https://admin-demo.nopcommerce.com/login");
Thread.sleep(3000);
PageFactory.initElements(driver, Login_pageObjects.class); // for null exception
Login_pageObjects.username.clear();
Login_pageObjects.username.sendKeys("admin@yourstore.com");
Login_pageObjects.password.clear();
Login_pageObjects.password.sendKeys("admin");
Login_pageObjects.loginButton.click();
}
Orange HRM PF Model
1.Page Objects class
public class OrangeHRM_PF_Objects {
WebDriver driver;
String URL="https://opensource-demo.orangehrmlive.com/web/index.php/auth/login";
@FindBy(how=How.NAME, using="username")
public static WebElement userName;
@FindBy(name="password")
public static WebElement passWord;
@FindBy(xpath="//button[@type='submit']")
public static WebElement logIn;
}
2.Test Case
public class OrangeHRM_PF_TestCase {
WebDriver driver;
String URL="https://opensource-demo.orangehrmlive.com/web/index.php/auth/login";
// ******************** browser **********************************
@Test(priority=1)
public void launch_Browser() {
System.setProperty("webdriver.chrome.driver","C:\\Users\\Reka\\Drivers\\chromedriver.exe");
driver=new ChromeDriver();
}
@Test(priority=2)
public void loginPage() throws InterruptedException {
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(10));
driver.get(URL);
PageFactory.initElements(driver, OrangeHRM_PF_Objects.class); // for null exception
OrangeHRM_PF_Objects.userName.sendKeys("Admin");
OrangeHRM_PF_Objects.passWord.sendKeys("admin123");
OrangeHRM_PF_Objects.logIn.click();
}
}
What does Maven stand for?
The Mars Atmosphere and Volatile Evolution (MAVEN) mission was selected as part of NASA's now-canceled Mars Scout Program to explore the atmosphere and ionosphere of the planet and how they interact with the Sun and solar wind.
What is POM XML in Selenium?
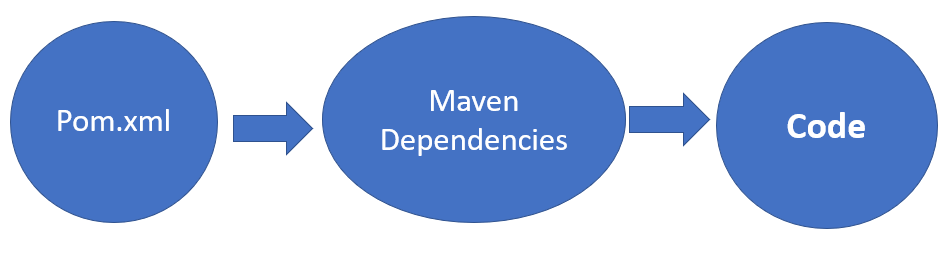
Maven project provide POM.xml file by default.
Maven project is controlled by pom.xml file
By default Maven project gives as project structure
Pom.xml will add dependencies and plugins
Generate Report
Document Project
Package the project
What does POM stand for?
POM is an acronym for Project Object Model. The pom. xml file contains information of project and configuration information for the maven to build the project such as dependencies, build directory, source directory, test source directory, plugin, goals etc.
It is a single configuration XML file called pom.xml that contains the majority of the information required to build a project.
The role of a POM file is to describe the project, manage dependencies, and declare configuration details that help Maven to build the project.
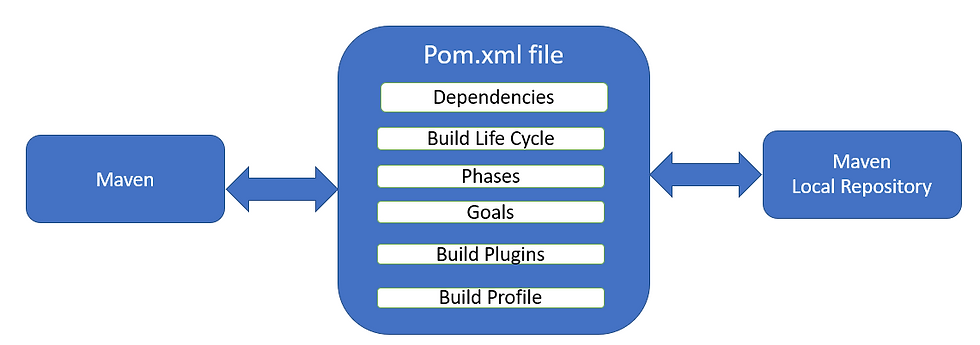
What is a Maven lifecycle?
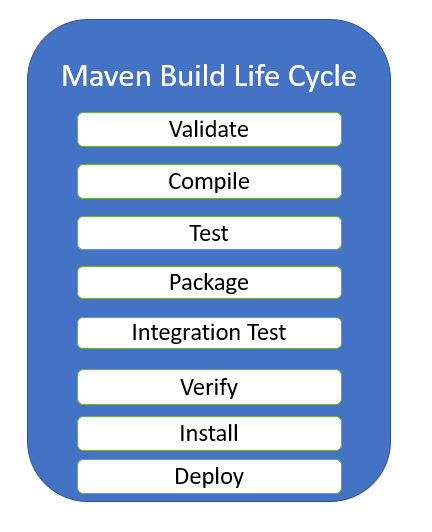
The maven builds lifecycle is the tasks performed when the maven build commands are executed. The maven build lifecycle is divided into stages known as build phases. A build phase is made up of objectives. Maven goals are specific tasks that contribute to the creation and management of a project.
Why POM xml is required?
A Project Object Model or POM is the fundamental unit of work in Maven. It is an XML file that contains information about the project and configuration details used by Maven to build the project. It contains default values for most projects.
Each Maven project has Pom.xml with default information about the project
<modelVersion>4.0.0</modelVersion>
<groupId>com.maven</groupId>
<artifactId>maven_training</artifactId>
<version>0.0.1-SNAPSHOT</version>
Why we need Maven dependencies?
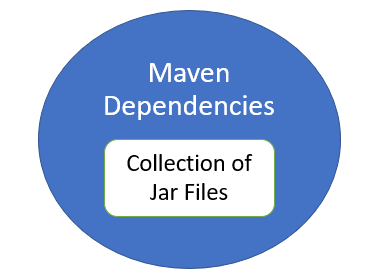
Maven is chiefly used for Java-based projects, helping to download dependencies, which refers to the libraries or JAR files. The tool helps get the right JAR files for each project as there may be different versions of separate packages.Dependencies are third-party software required by the project.
Why do we need to add dependency? Advantages of Dependency Management It helps when we switch from one version to another. It avoids mismatch of different versions . We only need to write a library name with specifying the version. It is helpful in multi-module projects.
Where does Maven get dependencies from? The Local Repository Maven's local repository is a directory on the local machine that stores all the project artifacts. When we execute a Maven build, Maven automatically downloads all the dependency jars into the local repository.
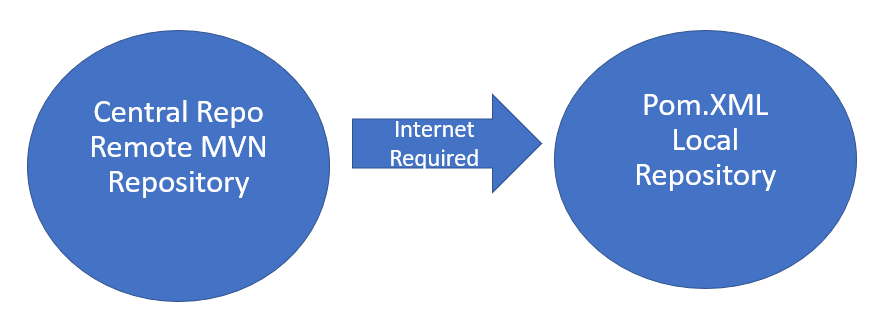
What is the difference between dependency and plugin? A plugin is an extension to Maven, something used to produce your artifact (maven-jar-plugin for an example, is used to, you guess it, make a jar out of your compiled classes and resources).
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.10.1</version>
</plugin>
A dependency is a library that is needed by the application you are building, at compile and/or test and/or runtime time.
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.6.0</version>
</dependency>
The external jar files that are needed for the project need to be added as dependencies in this pom file. So for example, if you need Selenium JAR files in your project, you will need to add Selenium dependencies in the pom file. Maven then automatically downloads the jar files and adds them to the classpath.
Conclusion:
I hope, This article will help you to understand about types of Framework, How to use Page Objects and Page Factory in Framework and the use of Maven Project pom.xml and their dependencies.
You must have got an idea on the topics explained in this blog. Lets explore more and learn New Topics.
Happy Learning