Code reusability is an integral part of efficient software development. It is true in case of developing automation framework as well. Just like in any other tool, automation in Postman involves writing scripts and tests to validate responses for structured request-flows. Features like variables (environment, global, collection and data), pre and post request scripts, folders inside collection, request chaining, etc. all help to facilitate effective ways to design code reusability in Postman. In this two part blog, we will explore a bit into JavaScript functions, package library and API workflow to achieve this goal.
To illustrate this concept with an example, we will be using the sample collection below. As shown in the diagram below (left), this collection consists of APIs that deals with creation of resources called Users. With the given sequence of APIs, the goal is to check how many users are already there to start with, and then create user (one with all fields or another with just mandatory ones), ensuring it could be retrieved using ID or first name, thereafter, making update to the created user and finally deleting it. On the right is a sample response of a PUT/POST or single User GET call.
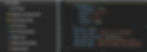
From validation point of view, there would be some tests that could be common across all requests and some would be only valid for either all GET calls or POST/PUT calls. For simplicity’s sake, we will start with very basic tests like header presence/value test, response time test and response structure test.
Instead of writing those test codes in each of the collection request’s Post-Response Script over and over again, we will write them at Collection level so it is accessible to all requests in the collection after execution of a request and a response receipt. For a quick refresher, here is a diagram from official Postman learning center of the “Execution order of scripts”:
Method 1: Test re-usability using Arrow Function Expression and Eval() function
Code explanation
Four separate “pm.test()” were created for four different validations and clustered under an anonymous (function that does not have any name associated with it) arrow function. This function is then assigned to a variable named “commonTests”.
Line 32: To execute this function, this variable needs to be called from the script. To make the variable accessible, it is set at collection level using “pm.collectionVariables.set(variablename)” method. We have to pass function variable name in the form of String using “toString()” method as Postman does not directly support passing entire functions between scripts.
Note: collection variable name and function variable name are kept the same.
Calling the method from the request script
Code explanation
Line 9: From the target request’s Post-response script, “pm.collectionVariables.get()” method is called to retrieve collection variable name. However, this alone won’t do. We need a function name "eval()" to evaluate the contents that have been written in a string so that the code is executed again.
However, there are some drawbacks to using eval() function. It can open up code to security vulnerabilities, especially if the input to eval() is not properly sanitized. It executes the code passed to it as a string, which can include arbitrary JavaScript code. If this input comes from user input or an untrusted source, it could possibly lead to code injection attacks.
Hence, we move on to next option which is using JavaScript Object.
Method 2: Test re-usability using Object function
Code explanation
As seen in the code above, we are now working with three tests. Response header related tests are clumped together under “postPutHeaderTest” method and response time test is written under another function name “postPutCommonTest”. Both these functions have been comma separated as they are assigned as properties of object “commonTests”.
Calling the method from the request script
Code explanation
Line 11 & 12: From the request level, all we have to do is call the object name followed by the function name. As seen in Test Results tab, all three tests executed in the order they were called.
This is the easier way to implement code re-usability and many more common tests could be written at Collection level. However, this will heavily clutter the Post script if written together with other things (like calling another request using “pm.execution.setNextRequest()”or “pm.sendRequest()” method). It would be much efficient to maintain these tests in some util class where common tests or other scripts could be kept as a repository. For that, Postman offers Package Library supports JavaScript code.
Method 3: Test re-usability using Package Library
Package Library can be used to store commonly used scripts and tests as packages in Postman team which facilitates maintaining scripts and tests in one location, and reusing them in personal, private, and team workspaces. Postman teammates can also access and import packages from the Package Library, enabling all to share scripts and tests directly in Postman.
Now let’s see how to create one.
First highlight all the lines of re-useable code. Immediately on the right side, “+ Add to package” option will appear in blue. Click on it and select “New Package”.
Code explanation
There it will be prompted to add a package name and summary (optional) while the package will be populated with code previously highlighted. In this example, only two postman tests are used and clustered under a function with name (unlike anonymous function written in first method).
Line 15-17: Since this package needs to be imported in the target post-res script, we have to export the function “commonTest” with the syntax shown to give its visibility throughout the collection.
Importing Package and Calling method from the request script
Code explanation
Line 1: From the script, the first thing that needs to be done is to import the package and for that we will need “pm.require(package name)” method which then needs to be stored in a variable:
const usercollectionReusabletests = pm.require('usercollection_reusabletests');
Note: If you are not sure of the syntax of importing a package, then click on the “Packages” box on the right side in snippet section. It will show the list of available packages. Click on the one you want to import and the line above will auto-populate your script
Line 4: Access the method “commonTest()” by calling the package variable name usercollectionReusabletests followed by function name.
Now that we have seen how package works, let’s refactor the code a bit.
Code explanation
More tests were added inside the same function like common tests for POST/PUT calls and for any kind of HTTP calls. Call type and corresponding test name for that call type could be passed as function arguments from the request script as needed.
In the collection, there are two POST calls with different sets of payload data (one where both mandatory and optional fields have data and another has only mandatory fields populated). Both the scripts will share almost similar common test validations. Similarly, in PUT call, where the created user will have some updated data in payload, the response will again have similar set of response validation as POST call. In this example, listed below are few tests that will be executed for both POST and PUT:
Validate status
Test response time
Test response header
Test response structure -- mandatory fields
Test response structure -- optional fields
Test mandatory field values
Test optional field values
Since status code for POST and PUT calls are different, they have been independently called from snippets without having to maintaining them in any package. For the rest of the tests, all it needed was to call the tests from package. Code now looks much neater and organized than having more than 100 plus lines of codes in each of script for each type of requests to achieve the same goal.
All we need now is to run the collection and all these tests will start executing one by one for the respective call types in the collection. More on request chaining to manipulate execution workflow is explored in Part 2.
Git
Reference
Postman Learning Center, Reuse scripts in Postman. Retrieved from
This webpage introduces the concept of package library and its usage
Postman Learning Center, Use scripts to add logic and tests to Postman requests in Postman. Retrieved from
This webpage discusses the execution order of scripts
How to reuse Postman scripts and tests among different test cases. Retrieved from
This YouTube video discusses eval() method offering valuable insights for understanding scripts and tests reusability