Introduction
The Stream API, introduced in Java 8, is a powerful extension of the Collections API. It enables developers to work with sequences of elements and perform various operations such as filtering, sorting, mapping, and collecting data from collections. By combining these operations, developers can create a pipeline to efficiently query and process data in a more streamlined and functional manner.
What is Stream API?
Stream API represents a sequence of elements that can be processed sequentially or in parallel. Unlike traditional collections, a stream does not store data; instead, it operates on the data in the underlying collection without modifying it. The Stream API promotes functional programming standard by supporting operations as lambda expressions or method references, resulting in more readable and maintainable code. Overall, the Stream API in Java provides a powerful and efficient when working with collections of data.
Why Stream is called as API?
The Stream API in Java is called an API because it provides a well-defined interface and set of methods that allow developers to work with streams of data in a consistent and standardized way.
An overview of How Java Streams Work:
The simplest way to understand the Java streams is to imagine a list of objects disconnected from each other and entering a pipeline one at a time. You can control the number of objects which are entering the pipeline, function which undergo inside it, and how you catch them when they exit the pipeline.
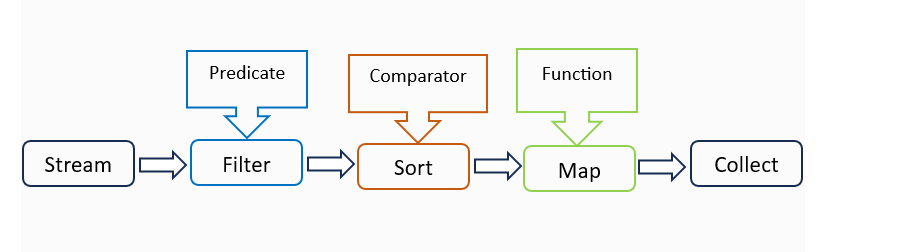
Predicate:
Predicate is a functional interface used to filter elements in a stream. It acts as a condition or criteria that determines whether an element should be included in the resulting stream or not.
Comparator:
Comparator is a functional interface used for sorting elements in a stream. It provides a way to define the ordering of elements based on specific criteria.
Function:
Function is a functional interface used for transforming elements in a stream. It allows you to define a transformation logic that takes an input element and produces an output element of a potentially different type.
Different Operations On Streams
1. map: stream.map()
Stream provides a map() method to map the elements of a stream into another new object.
// 1. demonstration of map method
List<Integer> number = Arrays.asList(2, 3, 4, 6);
List <Integer> SqOfNum= number.stream().map(x -> x*x).collect(Collectors.toList());
System.out.println("The square of each number in the arrays are "+ SqOfNum + " respectively");
Output
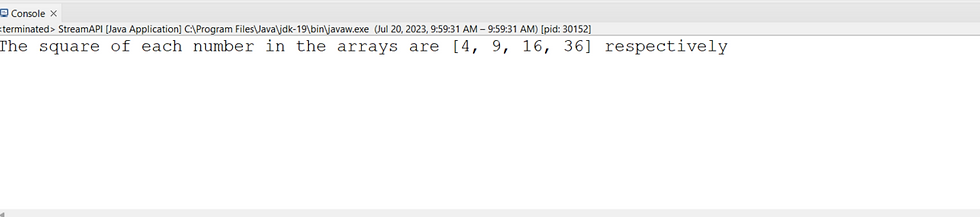
2. filter: stream.Filter()
The filter() method is used to filter a stream.
// create a list of String
List<String> modules = Arrays.asList( "Assignment Submission", "Assignment", "Batch", "User");
// demonstration of filter method
List<String> filtermodules= modules.stream().filter(s -> s.startsWith("A")).collect(Collectors.toList());
System.out.println("Filtered modules are "+filtermodules);
Output

3. sorted: stream.sort()
The sorted method is used to sort the stream.
// demonstration of sorted method
List<String>show=modules.stream().sorted().collect(Collectors.toList());
System.out.println(“Sorted Alphabetically ”+ show);
Output
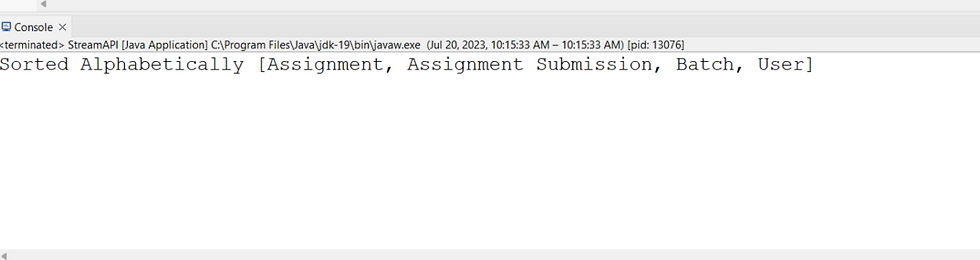
Terminal Operations
Terminal operations are essential for obtaining the final results of data processing in a stream. Some common terminal operations in the Stream API include:
collect()
Collects elements from the stream into a collection or a single value. It is often used to gather elements into a list, set, or map.
forEach()
Iterates through the elements of the stream and performs an action on each element. It is useful for performing side effects on the elements.
reduce()
Combines elements of the stream into a single result using a binary operator. It is used for tasks like summing, finding the maximum or minimum, or any other form of data reduction.
min() and max()
Returns the minimum and maximum elements of the stream based on a comparator.
count()
Returns the number of elements in the stream.
anyMatch(), allMatch(), and noneMatch()
Checks if any, all, or none of the elements in the stream satisfy a given condition (predicate).
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Terminal operations
long count = numbers.stream().count();
int sum = numbers.stream().reduce(0, Integer::sum);
int max = numbers.stream().max(Integer::compare).orElse(0);
boolean anyMatch = numbers.stream().anyMatch(num -> num > 3);
System.out.println("Count: " + count); // Output: Count: 5
System.out.println("Sum: " + sum); // Output: Sum: 15
System.out.println("Max: " + max); // Output: Max: 5
System.out.println("Any match > 3: " + anyMatch); // Output: Any match > 3: true

Method Chaining
Method chaining is a powerful feature in the Stream API in Java, where multiple methods are called on the same object in a single line of code. This allows for a more concise and fluent style of coding. Each method call returns the object on which the method was invoked, enabling subsequent methods to be called on the same object one after another.
// demonstration of one another method Chaining in Stream()
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 1
long count = numbers.stream()
.filter(num -> num % 2 == 0) // Keep only even numbers
.map(num -> num * num) // Square each even number
.skip(2) // Skip the first two squared numbers
.limit(3) // Limit to the next three squared numbers
.distinct() // Remove duplicates
.count(); // Count the remaining squared numbers
System.out.println(count); // Output: 3
Output
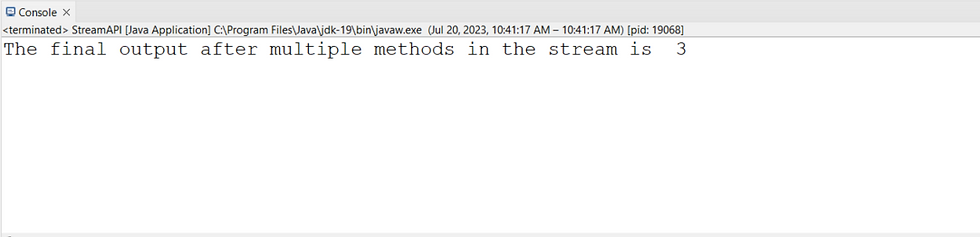
Conclusion:
Overall, the Stream API simplifies data processing tasks, improves code readability, encourages functional programming, and enables parallel processing, making it a valuable addition to Java programming for efficient and expressive data manipulation. Happy learning!!