What is Wait in Selenium
Selenium Wait is a set of commands that wait for a specified period of time before executing test scripts on the elements. Wait plays a very important role in executing test scripts. Lets see why we need wait commands in Selenium.
Why do we need Waits in Selenium?
When a page is loaded by the browser the elements which we want to interact with may load at different time intervals. Wait commands are essential for executing test scripts and help identify and resolve issues related to the time lag in doing particular action on web element. For example it is very common for testers to get the message “ElementNotVisibleException“. This appears when a particular web element with which WebDriver has to interact, is delayed in its loading .To resolve this issue we have to use wait commands in selenium.
Type of Selenium Waits
Selenium WebDriver provides two types of waits to handle synchronization issues.
Implicit Wait
Explicit Wait
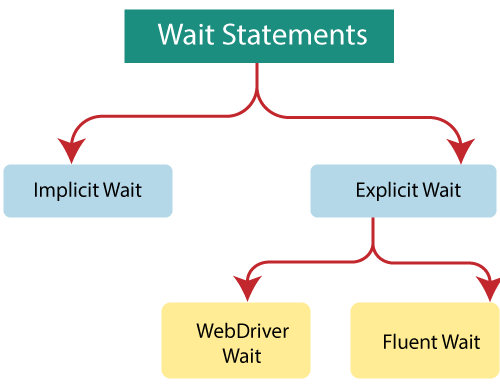
Implicit Wait in Selenium
Implicit wait allows you to halt the webdriver for a particular period of time until the webdriver locates an element on the web page. Implicit wait is applied globally, which means in your script you only need to write it one time and it will be applicable to all the web elements specified in the script.
The key point here is ,it will not wait for the complete duration of time mentioned, if it finds the element before specified time it will move to execute the next line of code .This will reduce the time of script execution and also helps in handling issues like “ElementNotVisibleException“ .Below syntax can be used for implicit wait.
To be able to access and apply implicit wait in our test scripts, we have to first import:
import java.time.Duration;
Implicit Wait syntax:
driver.manage().timeouts().implicitlyWait(Duration duration);
The implicit wait mandates to pass two values as parameters. The first argument indicates the time measurement scale . The second argument indicates the time in the numeric digits that the system needs to wait. Thus, in the below code, we have mentioned the “5” seconds as default wait time and the time unit has been set to “seconds”. You can select the duration as per your script requirement.
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(5));
Explicit Wait in Selenium
Explicit wait is condition based wait statement .The explicit wait is used to wait for specific conditions or the maximum time exceeded before throwing an "ElementNotVisibleException".
Once we declare explicit wait we have to use “ExpectedConditions”.In a scenario where you don't know the amount of wait time , you can use explicit wait like elementToBeClickable() or textToBePresentInElement().
Explicit wait is more intelligent, but can only be applied for specified elements and you need to do this for case by case. The more intelligent and optimized for timing in comparison with implicit wait and has its own price with “unnecessary” code everywhere in your automation scripts.
There are two main ways to implement Explicit Waits
WebDriverWait
FluentWait
WebDriverWait
WebDriverWait specifies the condition and time for which the WebDriver needs to wait. To apply WebDriverWait in our script we have to import :-
import java.time.Duration;
import org.openqa.selenium.support.ui.WebDriverWait;
import org.openqa.selenium.support.ui.ExpectedConditions;
WebDriverWait syntax:
WebDriverWait wait = new WebDriverWait(driver,Duration.ofSeconds(10));
We create a reference variable “wait” for WebDriverWait class and instantiate it using WebDriver instance and maximum wait time “10” Seconds for the execution to layoff.
Expected Condition:
wait.until(ExpectedConditions.elementToBeClickable
(By.xpath("//div[@class='card custom-card']//a")));
we use the “wait” reference variable of WebDriverWait class created in the previous step with ExpectedConditions class and an actual condition which is expected to occur. As soon as the expected condition occurs, it will move to execute the next line of code instead of forcefully waiting for the entire 10 seconds.
Explicit wait provides the following Expected conditions for usage:
alertIsPresent()
elementSelectionStateToBe()
elementToBeClickable()
elementToBeSelected()
frameToBeAvaliableAndSwitchToIt()
invisibilityOfTheElementLocated()
invisibilityOfElementWithText()
presenceOfAllElementsLocatedBy()
presenceOfElementLocated()
textToBePresentInElement()
textToBePresentInElementLocated()
textToBePresentInElementValue()
titleIs()
titleContains()
visibilityOf()
visibilityOfAllElements()
visibilityOfAllElementsLocatedBy()
visibilityOfElementLocated()
Fluent Wait
The Fluent wait is similar to explicit wait in terms of function. In this Wait, you can perform wait for action for an element only when you are unaware of the time it might take to be clickable or visible.
It also defines how frequently WebDriver will check if the condition appears before throwing the “ElementNotVisibleException”.
Fluent wait have two different factors.
Polling Frequency:-
Using Fluent wait you can change polling frequency based on your case requirement. That means you can define in your script to keep checking on an element after every "given" Seconds.
Ignore Exception:-
During polling, in case you do not find an element ,you can ignore any exception.
Import Statements:-
import org.openqa.selenium.ElementClickInterceptedException;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.ui.FluentWait;
import org.openqa.selenium.support.ui.Wait;
import java.time.Duration;
Fluent Wait syntax:
Wait<WebDriver> wait = new FluentWait<WebDriver>(driver)
.withTimeout(Duration.ofSeconds(10))
.pollingEvery(Duration.ofSeconds(2))
.ignoring(ElementClickInterceptedException.class);
Comparison
Implicit Wait | Explicit Wait |
Implicit wait is applied globally, which make it fast and easy to implement | It is Applicable to only certain element which is specific to a certain condition. |
In Implicit Wait, we need not specify “ExpectedConditions” on the element to be located. | In Explicit Wait, we need to specify “ExpectedConditions” on the element to be located |
Most effective when used in a test case in which the elements are located with the time frame specified in implicit wait | Most effective when the elements are taking a long time to load. Also useful for verifying property of the element such as visibilityOfElementLocated, elementToBeClickable,elementToBeSelected |
Conclusion:
Implicit, Explicit and Fluent Wait are the different waits used in Selenium. Usage of these waits are totally based on the elements which are loaded at different intervals of time.
Not a good practice to use :
Both implicit and explicit wait on same driver
Thread.sleep()