In this Blog we will write a Python code for
Login to a webpage
Run the code
Validate Actual output and Expected output
Pytest Assertion
Captured screenshot for failed first test case by giving wrong password
Skipping second test case
Passing third test case
Generate Allure report
Have mentioned the type of Severity at class level and method level
Python has a simple syntax similar to the English language. Python has syntax that allows developers to write programs with fewer lines than some other programming languages. Python runs on an interpreter system, meaning that code can be executed as soon as it is written. This means that prototyping can be very quick.
Top 8 Python Testing Frameworks
Behave Framework.
Lettuce Framework.
Robot Framework.
Pytest Framework.
TestProject Framework.
PyUnit (Unittest) Framework.
Testify Framework.
Doctest Framework.
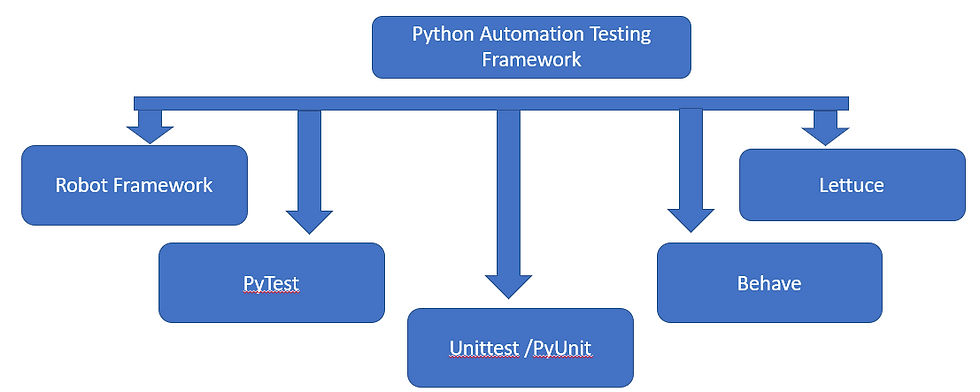
In this blog we will be using Pytest
Pytest is a Python testing framework that originated from the PyPy project. It can be used to write various types of software tests, including unit tests, integration tests, end-to-end tests, and functional tests. Its features include parametrized testing, fixtures, and assert re-writing.
Pytest a automation framework?
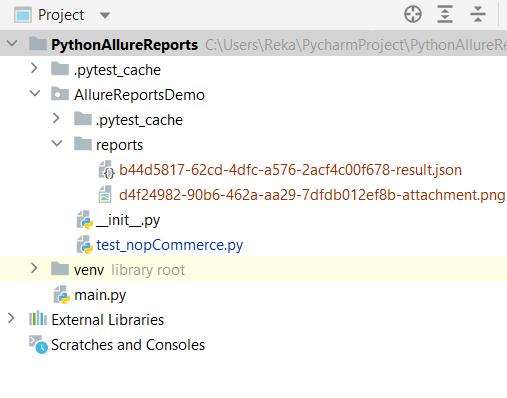
Pytest is one of the best open-source, simple, scalable and Python-based Test Automation Framework available at the market today. Pytest powers you to test anything including Databases, UI and is more popular among testers for API Testing.
Pytest is a test automation framework that allows developers and testers to write test scripts using Python language.
With pytest, this is the command-line tool called pytest . When pytest is run, it will search all directories below where it was called, find all of the Python files in these directories whose names start or end with test , import them, and run all of the functions and classes whose names start with test or Test .
What is severity in allure report?
Allure supports next severity levels: TRIVIAL , MINOR , NORMAL , CRITICAL , BLOCKER . By default, all tests marks with NORMAL severity.
@Severity In allure, we can define any @Test with @Severity annotation with any of these values like BLOCKER, CRITICAL, NORMAL, MINOR, TRIVIAL. By looking at this, we can understand the Severity of test if Failed.
You can add severity to your allure report by @severity decorator.
In this code , there are 3 test case
test_login
test_productlist
test_dashboardpage
Have mentioned 3 types of severity level NORMAL, MINOR and BLOCKER
Normal : Here i am skipping a test case to be implemented further
Blocker: If you are not able to login , and login is failing
Minor: If any Logo or color ... is not as per the requirements
What is assertion in pytest? The assert keyword is used when debugging code. The assert keyword lets you test if a condition in your code returns True, if not, the program will raise an AssertionError. You can write a message to be written if the code returns False.
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
import allure
import pytest
from selenium.webdriver.common.by import By
from allure_commons.types import AttachmentType
@allure.severity(allure.severity_level.NORMAL) # decorators class level
class TestNOP():
@allure.severity(allure.severity_level.BLOCKER) # decorator method level
def test_login(self):
serv_object = Service("C://Users//Reka//Drivers//chromedriver.exe")
self.driver = webdriver.Chrome(service=serv_object)
self.driver.implicitly_wait(5)
self.driver.get("https://admin-demo.nopcommerce.com/")
self.driver.find_element(By.XPATH,"//input[@id='Email']").clear()
self.driver.find_element(By.XPATH,"//input[@id='Email']").send_keys("admin@yourstore.com")
self.driver.find_element(By.XPATH,"//input[@id='Password']").clear()
self.driver.find_element(By.XPATH,"//input[@id='Password']").send_keys("admi")
self.driver.find_element(By.XPATH,"//button[normalize-space()='Log in']").click()
actual_title=self.driver.title
if actual_title=="Dashboard / nopCommerce administration":
self.driver.close()
assert True
else:
# capture screenshot and part of allure report
allure.attach(self.driver.get_screenshot_as_png(),name="testLoginScreen",attachment_type=AttachmentType.PNG)
self.driver.close()
assert False
@allure.severity(allure.severity_level.NORMAL)
def test_productlist(self):
pytest.skip("skipping test later it will be implemented")
@allure.severity(allure.severity_level.MINOR)
def test_dashboardpage(self):
serv_object = Service("C://Users//Reka//Drivers//chromedriver.exe")
self.driver = webdriver.Chrome(service=serv_object)
self.driver.get("https://admin-demo.nopcommerce.com/")
self.driver.find_element(By.XPATH,"//input[@id='Email']").clear()
self.driver.find_element(By.XPATH,"//input[@id='Email']").send_keys("admin@yourstore.com")
self.driver.find_element(By.XPATH,"//input[@id='Password']").clear()
self.driver.find_element(By.XPATH,"//input[@id='Password']").send_keys("admin")
self.driver.find_element(By.XPATH,"//button[normalize-space()='Log in']").click()
status = self.driver.find_element(By.XPATH, "//img[@class='brand-image-xl logo-xl']").is_displayed()
if status == True:
assert True # pytest assertion
else:
assert False
self.driver.close()
Pytest is a software test framework, which means pytest is a command-line tool that automatically finds tests you've written, runs the tests, and reports the results.
To execute the code , you have to run the following commands in Terminal .
-v
flag controls the verbosity of pytest output in various aspects: test session progress, assertion details when tests fail, fixtures details with --fixtures , etc.
Passing -v at least once is that the test names are output one per line as they run
pytest normally appends a message to the failure text
Notice that: Each test inside the file is shown by a single character in the output:
. for passing
F for failure.

-s
By default pytest captures standard output while running tests. If a test fails ,
it shows the captured output.

# Execute the code in terminal:
pytest -v -s AllureReportsDemo/test_nopCommerce.py
# Execute the code and save json format report in reports folder:
json report and screenshot will be stored in reports folder , once u run the below command
pytest -v -s --alluredir="C:\Users\Reka\PycharmProject\PythonAllureReports\AllureReportsDemo\reports"
# AllureReport generation
allure serve C:\Users\Reka\PycharmProject\PythonAllureReports\AllureReportsDemo\reports
Screenshot of the failed test case
I have given wrong password , so it should display credentials provided are incorrect
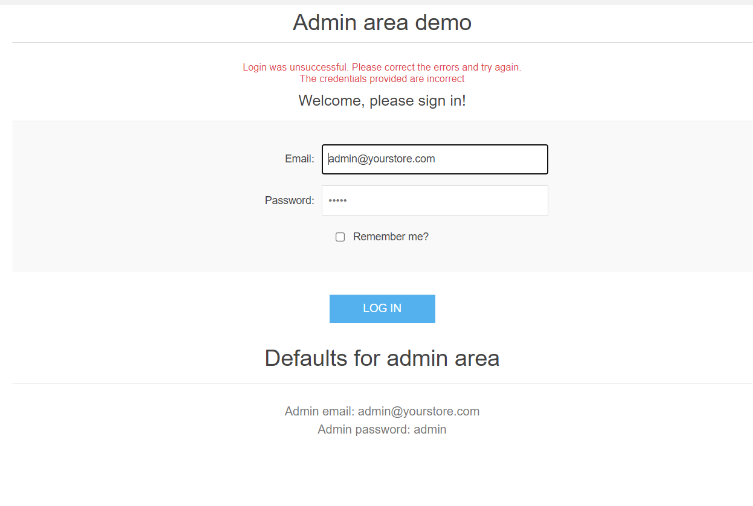
What is an allure report in Pytest? Allure Report is a flexible, lightweight multi-language test reporting tool. It provides clear graphical reports and allows everyone involved in the development process to extract the maximum of information from the everyday testing process
Allure Report : It shows 3 test cases , with 1 passed , 1 failed and 1 skipped
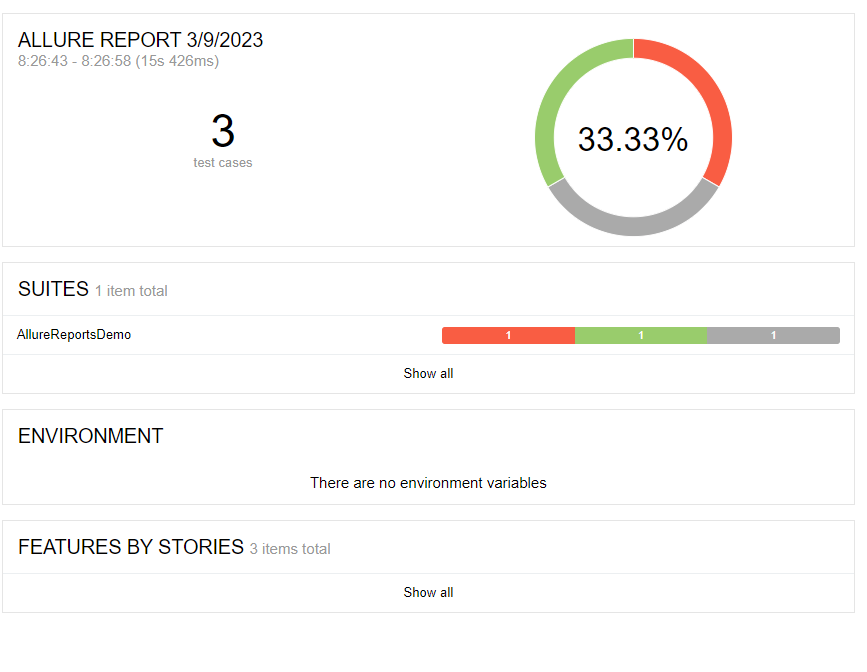
Conclusion:
I hope, This article will help you to understand How to generate Allure report in python pytest.
You must have got an idea on the topics explained in this blog. Lets explore more and learn New Topics.
Happy Learning