What is Maven?
Maven is a project management automation tool, and it is used for build management and dependencies. Java developers mainly use it. For testers, it helps to manage the dependencies which are .jar files. During build, the project or source code is converted into JAR files. It is used to compile source code, test, package and deploy it.
We need to follow these four steps to install Maven on our machine
1. Download MAVEN zip file & Extract
2. Setup MAVEN bin path
3. Set MAVEN_HOME
4. Verify MAVEN Installation
Step 1. Download MAVEN zip file & Extract
i) To download Maven, visit following website
ii) Click the Binary zip link from second row below

iii) After download is complete, extract the Maven archive to C:\Program Files
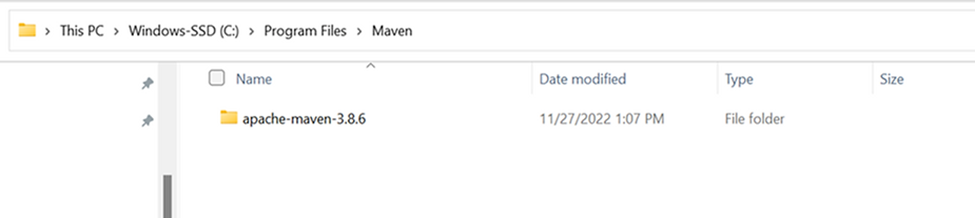
Open above apache-maven-3.8.6 folder
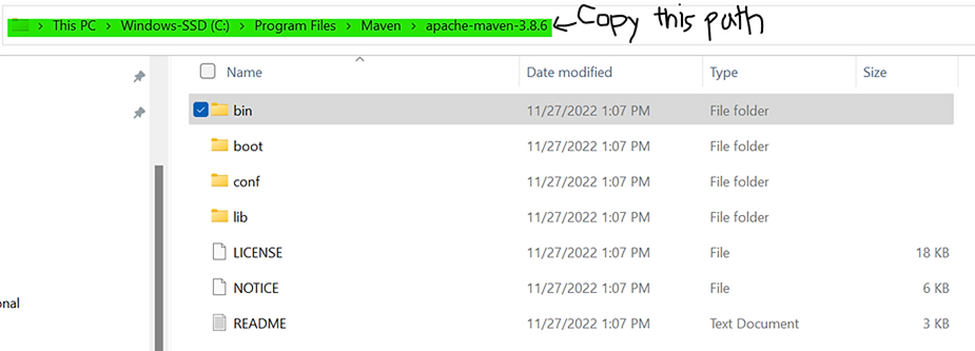
You can see bin folder is there, which is the important folder. Copy that path. Here the path link is ( C:\Program Files\Maven\apache-maven-3.8.6 ). Now we are going to set MAVEN bin path.
Step 2. Setup MAVEN bin path
i) Open Environmental Variables window from Start menu.
ii) Click the Edit the Systems Environment Variables option. Then System Properties window will open.
iii) Click on the Environment Variables tab.
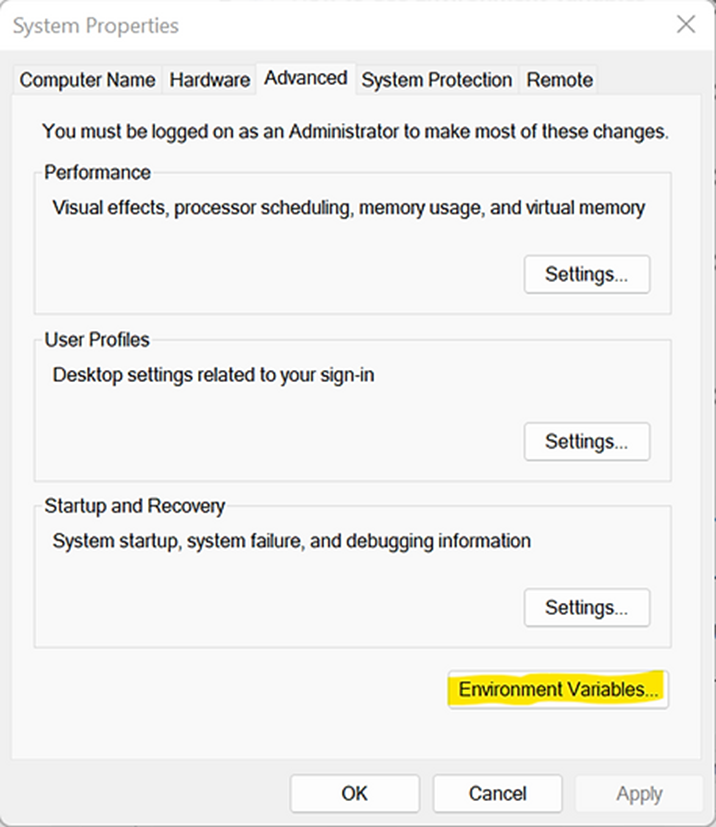
iv) Select Path option under the System Variables section. Click on Edit button.
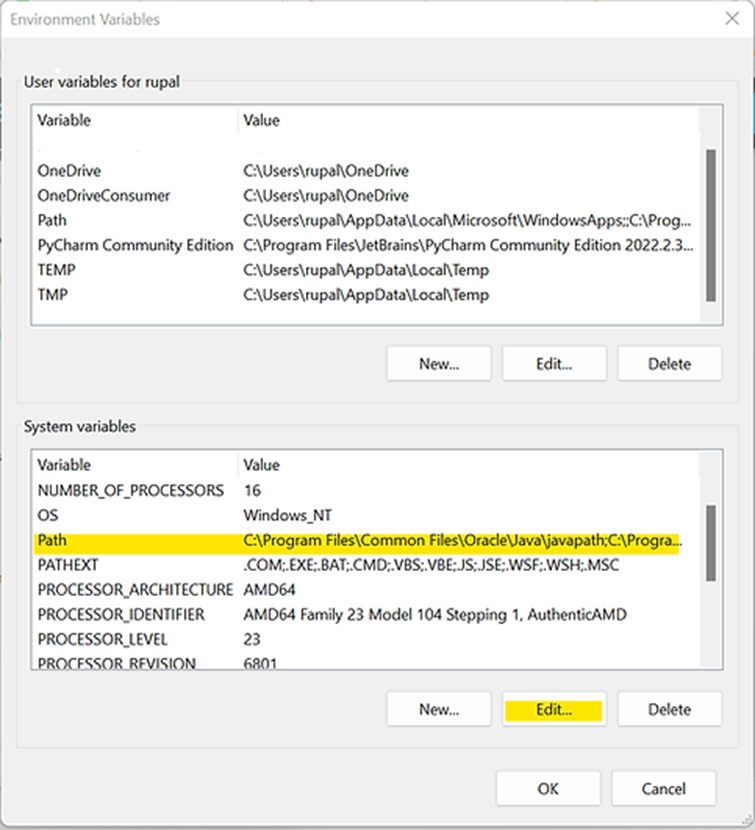
v) Click on New button.
- Paste the path to MAVEN bin folder which we have already copied in previous step.
( C:\Program Files\Maven\apache-maven-3.8.6 )
- Click OK button.
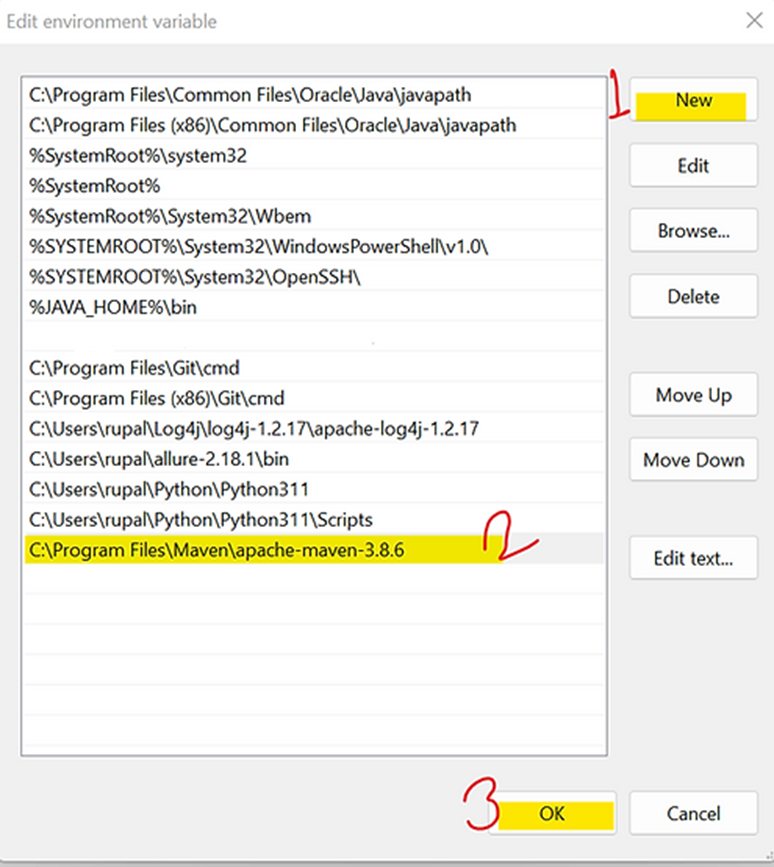
vi) Click OK button in Environment Variables window
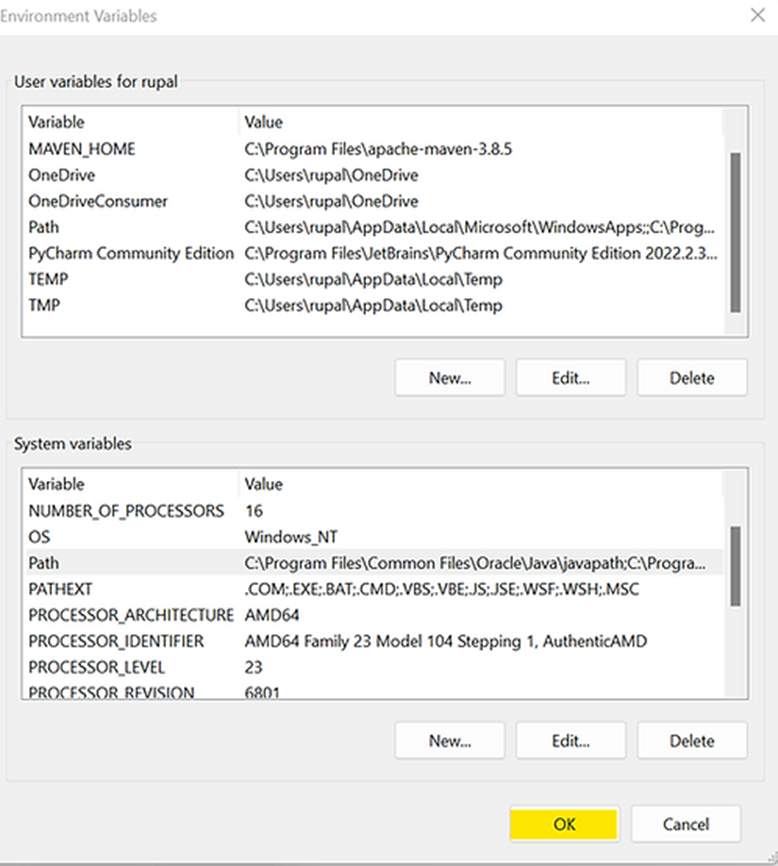
Now we are done with setting up MAVEN bin path. Next, we are going to set MAVEN_HOME.
Step 3. Set MAVEN_HOME
i) Click New button (highlighted) under the System Variables section to add new System Environment variable.
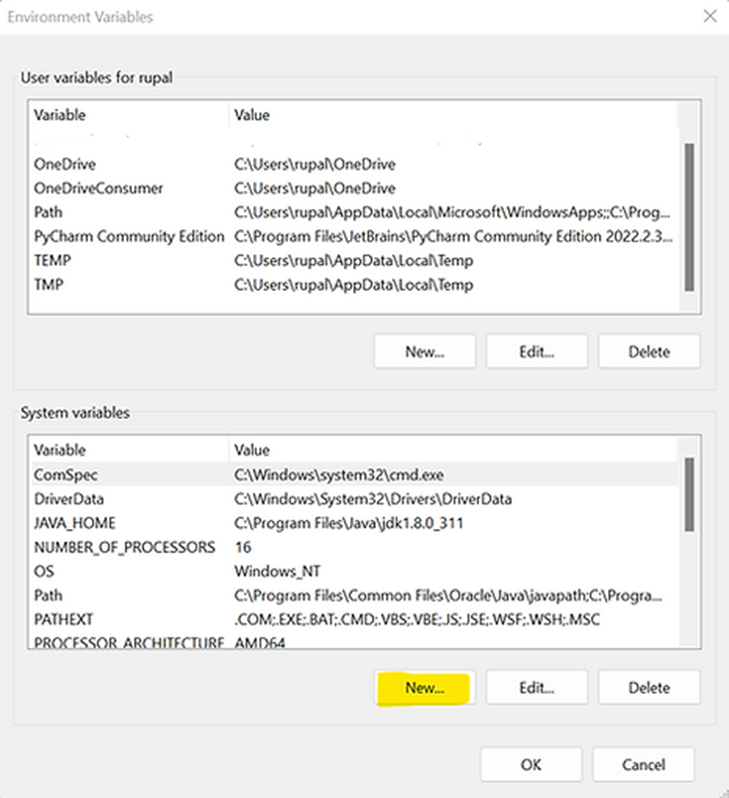
ii) Enter variable name as MAVEN_HOME.
Variable value is the path to the Maven bin folder (which we have already copied in previous steps C:\Program Files\Maven\apache-maven-3.8.6 ).
Click OK to save the new system variable.
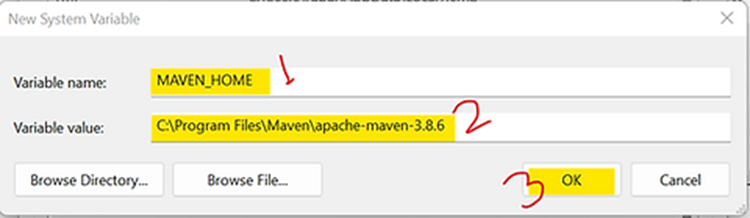
Step 4. Verify MAVEN Installation
In command prompt use the following command to check whether Maven is installed correctly.

It will show the version of Maven installed in your machine. So we are done with installing Maven successfully in our machine.
How to create a Maven project in Eclipse IDE
1. Open Eclipse IDE.
2. Click on File => New => Project
3. Click on the arrow near Maven, and it will show more options.
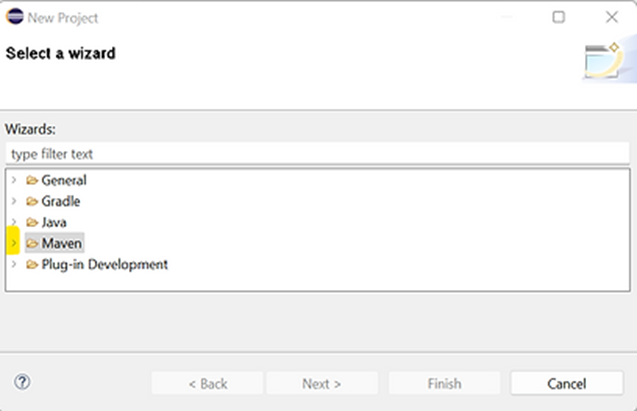
4. Select Maven Project option. Click on Next button.
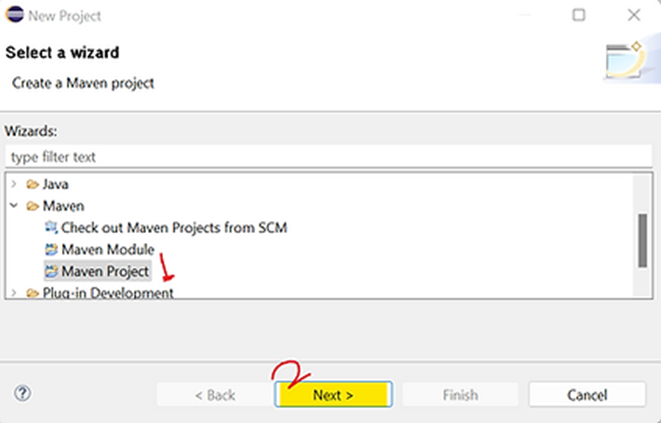
5. Select the check boxes as shown and click Next button.
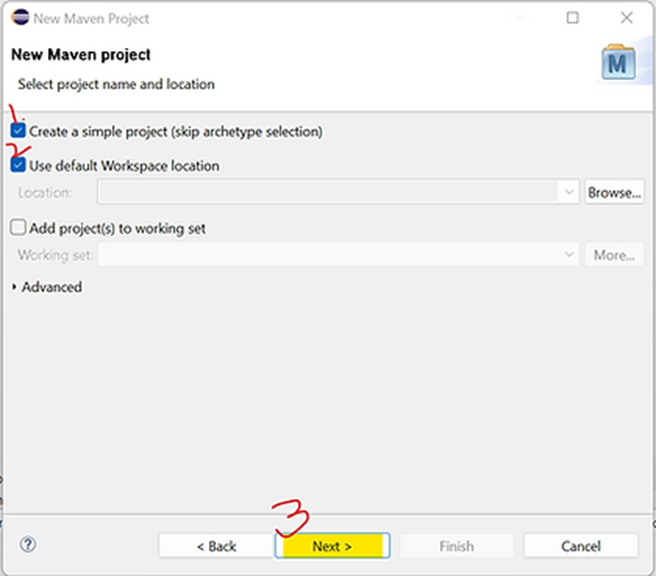
6. Fill in Group-Id, Artifact-Id and Name and click on Finish button.

7. We can see our project in Package Explorer.
Maven projects are configured using a POM (Project Object Model), which is stored in pom.xml file.
POM.xml
POM.xml stands for Project Object Model file and it is the main artifact of Maven. It is in the root of Maven project. Most projects depend on external jars to build and run correctly. Whenever you are testing any app or website using an automation tool, you need to download the required jars and add inside lib folder. Instead of doing it manually, if you use maven, POM.XML will be generated and it will automate the process of adding required jars for your project. For that you need to add required dependencies and plugins in pom.xml and then the jars you configured there will be added to your local repositories. It is an XML file that contains information about the project and configuration details used by Maven to build the project such as dependencies, build directory, source directory, goals, plugins etc.
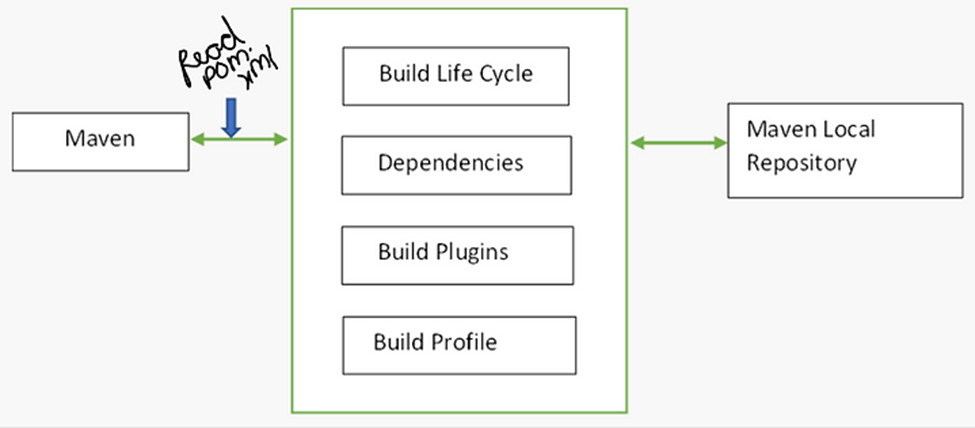
When executing a task or goal, Maven looks for the POM in the current directory. It reads the POM, gets the needed configuration information, then executes the goal.
Before creating POM, we should first decide the project group (group-id), it's name (artifact-id) and its version as these attributes help in uniquely identifying the project in repository.
POM.xml can be divided into three parts. First, Project meta data where project name, version etc. are present. Second, Dependencies which has the list of projects we depend on and third, plug-ins which is required for additional custom tasks to run.
Project coordinates are used to uniquely identify a project. It has three main elements (GAV):
1. Group ID: Name of the company or Organization which is used in reverse domain name (e.g., com.numpyninja)
2. Artifact ID: Name of the project
3. Version: A specific release version like 1.0. If the project is under active development then it is written as 1.0-SNAPSHOT else 1.0 FINAL.
Check the below screenshot for more understanding
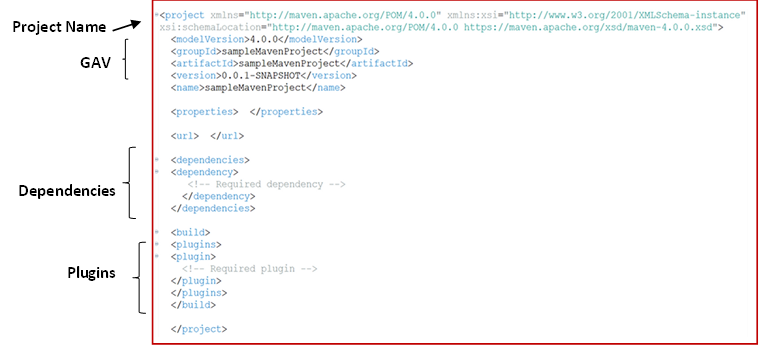
Whenever you created a Maven project, POM.XML gets created as shown below:
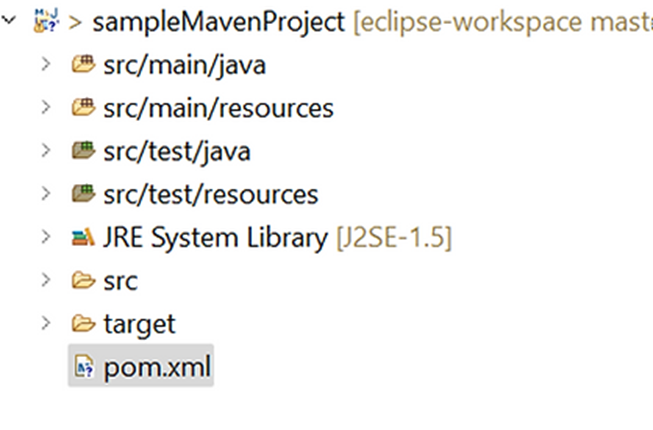
Build Lifecycle of Maven
When a maven command is executed, it goes through a process called the build lifecycle. There are three built-in build lifecycles in maven. They are:
1. Default: manages project build and deployment.
2. Clean: manages project cleaning. It deletes all the artifacts and files generated by the previous build and keeps the project clean.
3. Site: manages the creation of project site documentation.
Phases of Maven Build Lifecycle:
Each build lifecycle goes through a set of steps called 'phases'. The most common default lifecycle has the following phases:
· Validate - validate the project is correct and all necessary information is available.
· Compile - compile the source code of the project.
· Test - evaluate the compiled source code using a suitable unit testing framework. It is not required to be packaged or deployed.
· Package - take the compiled code and package it in its distributable format. It converts the whole project into a .jar file or .war file.
· Verify - run any checks on results of integration tests to ensure quality criteria are met.
· Install - install the package into the local repository for use of another project.
· Deploy - done in the build environment, copies the final package to the remote repository for sharing with other developers and projects. The build phases given above are executed in the same exact order. It is possible to specify which phase to run. For example, if we just want to compile the project and do not want to run the tests, we can specify the following command:

This will run the validate and compile phase.

If we specify then maven will run the validate, compile, test, package and verify phases. You should have realized that specifying a phase in the command will result in maven running all the other previous phases.
How Maven Works?
Maven working process can be easily understood by below flow diagram:
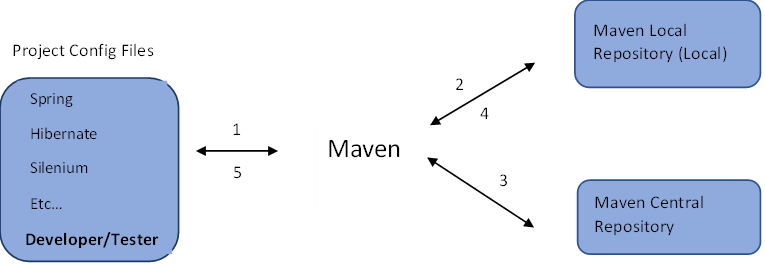
1. Maven read the config file given by developer/tester.
2. Maven checks the Maven Local Repository.
3. If files are not present in the Maven Local Repository, then Maven will go out to the internet at Maven Central Repository and pull those files from the Central Repository
4. Save those JAR files to the Local Repository to build up the local
5. Maven will use those JARS to build and run the application.
Problems solved by Maven
When building any JAVA project, additional JAR files are needed (e.g., Spring, Hibernate etc.). One approach to do so is to download the JAR files from each project website and then manually add the JAR files to the build path/class path. This is a tedious process and can cause error during the process of download and addition to the build path.
Maven is the friendly helper and the solution for the previously described problems. Just tell the Maven about the projects you are working with (e.g., Spring, hibernate etc.) and Maven will go out and download all the JAR files needed for those projects. Maven will make those files available during the compile or run time.
Standard Directory Structure provided by Maven and their Benefits
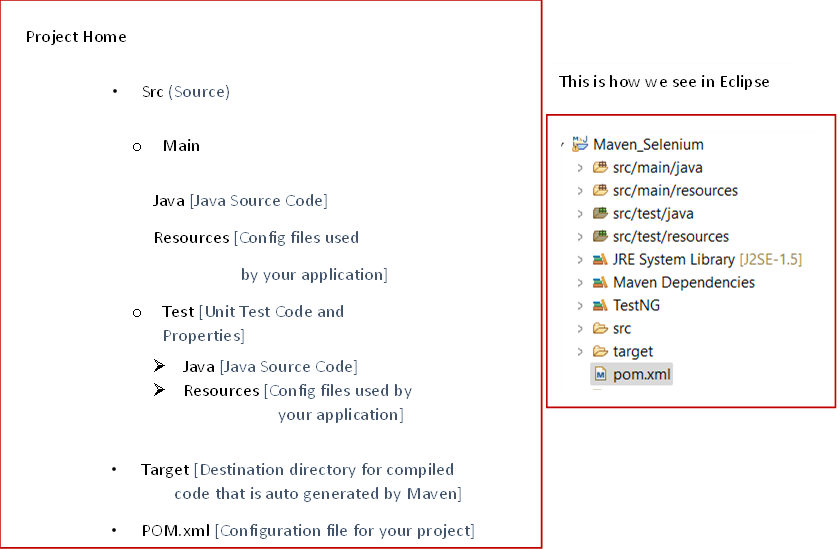
Few benefits of using Maven are:
1. For any new developers/Testers joining a project, they can easily find the code, properties, files, unit tests, etc. by looking at the Standard Directory Structure.
2. Most major IDEs (e.g., Eclipse, IntelliJ, NetBeans etc.) have built in support for Maven. So, IDEs can easily read and import Maven projects.
3. Helps manage all the processes, such as building, documentation, releasing, and distribution in project management.
4. Simplifies the process of project building.
5. Increases the performance of the project and the building process.
6. The task of downloading Jar files and other dependencies is done automatically.
7. Provides easy access to all the required information.
8. Makes it easy for the developer to build a project in different environments without worrying about the dependencies, processes, etc.
9. In Maven, it’s easy to add new dependencies by writing the dependency code in the pom. file conversely, Maven has a few drawbacks.
10. Maven requires installation in the working system and the Maven plug-in for the IDE.
11. If the Maven code for an existing dependency is unavailable, you cannot add that dependency using Maven itself.
Conclusion
Maven provides pom.xml, where we can manage dependencies easily. Dependency management in previous days was the most complicated mess for handling complex projects. The quick project set up is achieved through POM files. The way it works is that it allows you to define dependencies to external libraries that your project needs, and when you use Maven to build your project it will fetch these libraries from the web (external repositories) and add them to your built project. So, it's an automatic handling of dependencies.