In this blog we will be learning all the operations we can do in Array List and Linked List , this blog will be very useful for those want to learn Java Collections
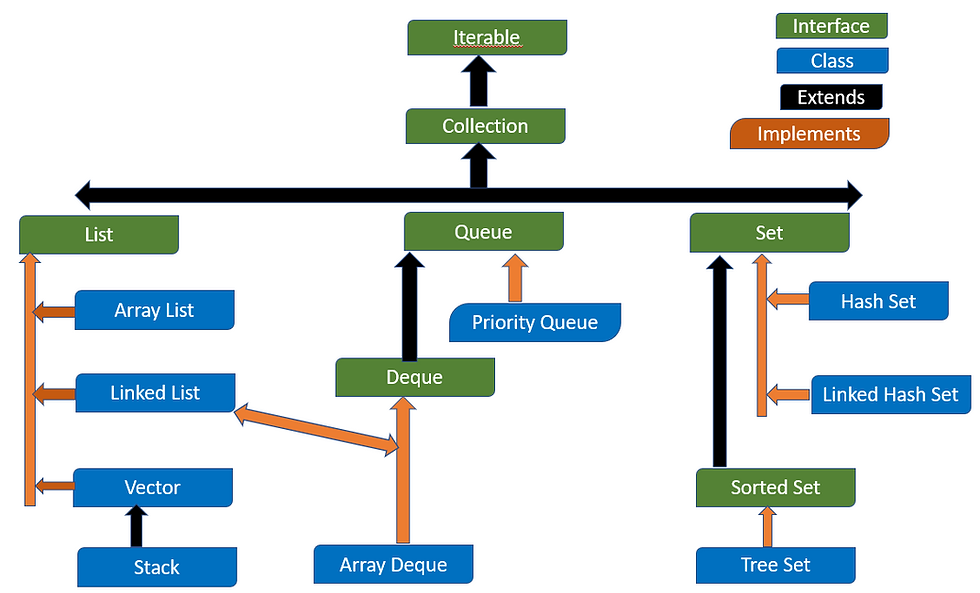
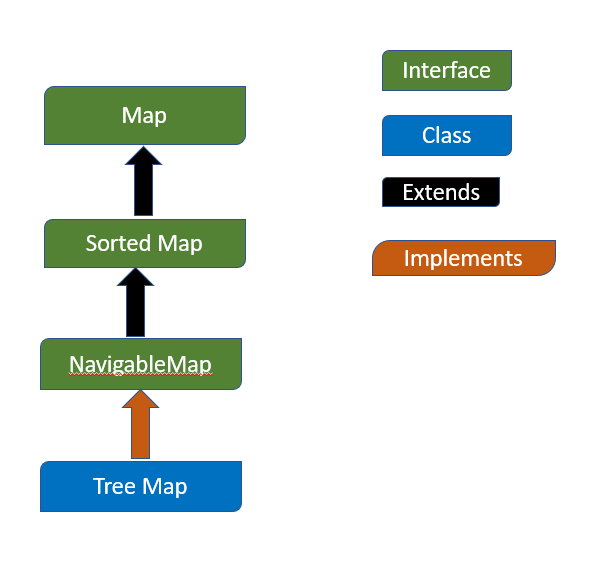
Array
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value.
To declare an array, define the variable type with square brackets.
Array indexes start with 0. [0] is the first element.
[1] is the second element, etc.
ArrayList
ArrayList provides us with dynamic arrays in Java.
List(I) is the Child of Collection(I). ArrayList (C) is one of the classes provides implementation for the List(I). In list duplicate values are allowed and the insertion order is maintained. The underlying DS is resizeable Array or Growable Array. We can insert Heterogeneous objects as well. NOTE: All the collections can store Heterogeneous objects can be stored except TREE SET and TREE MAP. ArraList and vector implements RandomAccess, Serializable and Cloneable Interfaces Synchronized-> No Thread safe-> NO Default capacity-10 Fill Ratio or Load factor:1 or 100% Growth Rate: current_size + current_size/2
package collections;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
public class ArrayListExample {
public void arrayListExample() {
// In arrayList insertion order is maintained
List<String> arrayList=new ArrayList<String>();
arrayList.add("Benz");
arrayList.add("Duggati");
arrayList.add("BMW");
arrayList.add("Bentley");
// allows duplicate
arrayList.add("Bentley");
System.out.println(arrayList);
System.out.println(arrayList.get(0));
System.out.println(arrayList.indexOf("Bentley"));
System.out.println(arrayList.lastIndexOf("Bentley"));
System.out.println(arrayList.indexOf("Maruti"));
// if that element is not in list , it returns -1
List<String> anotherList=new ArrayList<String>();
anotherList.addAll(arrayList);
// add elements from one list to another list
System.out.println(anotherList);
anotherList.clear();
System.out.println(anotherList);
//method overloading as same method can be run in two different ways by type of parameters(index or object) passing
arrayList.remove(0);
System.out.println(arrayList);
arrayList.remove("Bentley");
System.out.println(arrayList);
//null insertion is possible
arrayList.add(null);
System.out.println(arrayList);
//update ie change the value of the elmenet in particular position
arrayList.set(0, "chevrolet");
System.out.println(arrayList);
System.out.println(arrayList.isEmpty());
//Iterate the elemnets from the list
//using for each
for (String eachElement : arrayList) {
System.out.println(eachElement);
}
//using for loop
for(int i=1;i<arrayList.size();i++)
{
System.out.println(arrayList.get(i));
}
// forward traversing using List Iterator
ListIterator<String> list_iterator=arrayList.listIterator();
// ListIterator it is an interface , so u cant create object
while(list_iterator.hasNext())
{System.out.println(list_iterator.next());
}
// backward traversing using List Iterator
while(list_iterator.hasPrevious())
{System.out.println(list_iterator.previous());
}
// using iterator ONLY FORWARD direction is possible
Iterator<String> iterator=arrayList.iterator();
while(iterator.hasNext()) {
System.out.println(iterator.next());
}
// array list is not synchronised
//when u want to add element to an array when u iterate ie when u want to read , u also want to write , u have a class called CopyOnWriteArrayList
/* for (String eachElement : arrayList) {
System.out.println(eachElement);
arrayList.add("TATA"); // when u try to add to an array when u iterate (display) element from an array , u get Exception in thread "main" java.util.ConcurrentModificationException
}
*/
// can insert Heterogeneous objects(if generics are not used)
List hetero_arrayList=new ArrayList();
hetero_arrayList.add("Reka");
hetero_arrayList.add(15);
System.out.println(hetero_arrayList);
}
public static void main(String[] args)
{
ArrayListExample example=new ArrayListExample();
example.arrayListExample();
}
}
Linked List
LinkedList class is almost identical to the ArrayList. LinkedList class has all of the same methods as the ArrayList class because they both implement the List interface. LinkedList has a container inside. The list has a link to the first container and each container has a link to the next container in the list.
LinkedList is implemented using Doubly linked list. This is best suited for insertion and deletion operations.
Unlike ArrayList, this is not the best for retrieving the data.
All the collections implements Serializable and cloneable Interfaces.
LinkedList also implements those Interfaces but not RandomAccess Interface.
package collections;
import java.util.Iterator;
import java.util.LinkedList;
public class LinkedListExample {
public void linkedListExample() {
// create a simple Linked List
// insertion order is maintained
LinkedList<Integer> linkedList=new LinkedList<Integer>();
linkedList.add(2);
linkedList.add(3);
linkedList.add(4);
linkedList.add(4); // duplicate is allowed
linkedList.add(5);
System.out.println("Linked List :" +linkedList);
// add an element to the first position
linkedList.addFirst(1);
System.out.println("Linked List after adding first :" +linkedList);
// add an element to the last position
linkedList.addLast(6);
System.out.println("Linked List after adding last :" +linkedList);
// get the first value
System.out.println("first value :" +linkedList.getFirst());
// get the first value using index position
System.out.println("first value using index :" +linkedList.get(0));
// get the third value
System.out.println("Third value of the list using index :" +linkedList.get(3));
// remove first
System.out.println("Remove first : "+ linkedList.removeFirst());
System.out.println(linkedList);
// remove last
System.out.println("Remove first : "+ linkedList.removeLast());
System.out.println(linkedList);
//poll method and pollFirst() deletes the first element in the list
System.out.println(linkedList.poll());
System.out.println(linkedList);
//poll last deletes last element
System.out.println(linkedList.pollLast());
System.out.println(linkedList);
//remove deletes the first elemnet
linkedList.remove();
System.out.println(linkedList);
//Adding
linkedList.addFirst(1);
linkedList.add(2);
linkedList.add(3);
linkedList.add(4);
linkedList.add(5);
linkedList.add(6);
// remove First Occurence
linkedList.removeFirstOccurrence(2);
System.out.println("After removing the first occurence of 2" + linkedList);
linkedList.removeLastOccurrence(6);
System.out.println("After removing the last occurence of 6" + linkedList);
}
//Iteration of Linked List with simple for loop
public void iterateLinkedListWithSimpleFor()
{
LinkedList<String> linkedList=new LinkedList<String>();
linkedList.add("a");
linkedList.add("b");
linkedList.add("c");
linkedList.add("d");
//using for loop
for(int index=0;index<linkedList.size();index++) {
System.out.println(" Elements in the LL are " + linkedList.get(index));
}
//using advnced for loop(for each)
for (String string : linkedList) {
System.out.println(" Elements in the LL are "+ string);
}
// while loop
int number=0;
while(linkedList.size()>number) {
System.out.println(" Elements in the LL are " + linkedList.get(number));
number++;
}
// using Iterator
Iterator<String> iterator=linkedList.iterator();
while(iterator.hasNext()) {
System.out.println(" Elements in the LL are "+ iterator.next());
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
LinkedListExample example=new LinkedListExample() ;
//example.linkedListExample();
example.iterateLinkedListWithSimpleFor();
}
}
Conclusion:
I hope, This article will help you to understand ArrayList and LinkedList , how to add , remove , insert , update , delete, iterate .... Please Refer Part 2 and Part 3.
You must have got an idea on the topics explained in this blog. Lets explore more and learn New Topics.
Happy Learning