Java
· Invented by James Gosling and sun microsystems in 1991
· Object Oriented Programming Language
· High level language that is easy to read and understand
· Used in console, GUI, web app, mobile app and game development
· Used to develop software for devices
· Code can be written once and reuse everywhere
· Java uses Camel Casing naming conversion

Java Consists of 2 elements
1. Methods – Block of code that performs a task only when it’s called.
Example:
TV remote button it performs task when pressed.
2. Classes- A group of methods make classes. Mainly used to organize code.
Example:
Supermarket- fruits section, vegetable section, medical section etc. (consider each as a method)
Features of java
· Simple syntax. It is very easy to understand and learn.
· It is robust---it eliminates errors that occur in C/C++.
· It has garbage collectors and exception handling (this feature makes java very unique).
· Its popular because it is platform independent and it is portable (Program can be written somewhere and application is executed on different system/platform).
· Java can run on any machine and compilation takes place in byte code.
· Secure and has no virus.
· It has multithreading (Can perform multitasking)
· Datatype in java is set permanently (Fixed size of code allocation). If data type sizes were different across platforms, then cross-platform consistency is sacrificed.
· Its not as fast as c, C++ but it provides high performance than any other language.
Why Use Java?
Java works on different platforms (Windows, Mac, Linux, Raspberry Pi, etc.)
It is one of the most popular programming languages in the world
It has a large demand in the current job market
It is easy to learn and simple to use
It is open-source and free
It is secure, fast and powerful
It has huge community support (tens of millions of developers)
Java is an object oriented language which gives a clear structure to programs and allows code to be reused, lowering development costs
As Java is close to c++ and c# , it makes it easy for programmers to switch to Java or vice versa
4 Major concepts in Java
1. Abstraction- Showing only relevant information for a task to end user
2. Encapsulation- Binding of data and method into a class using private/protected access modifier. Data is stored within class and it’s not easy to access outside the class.
3. Inheritance-The attributes and characteristics of one class (Parent class) can be inherited by another class (child/sub class). Coders will take less time to reuse the code.

4. Polymorphism-Process of representing one form in multiple forms. Same method/variable/object will behave differently at different scenarios. Using same method for different task.
TIP:
Naming conversions
1. Camel Case - In camel case, you start a name with a small letter. If the name has multiple words, the later words will start with a capital letter
examples of camel case: firstName and lastName
2. Snake Case - Like in camel case, you start the name with a small letter in snake case. If the name has multiple words, the later words will start with small letters and you use an underscore (_) to separate the words
examples of snake case: first_name and last_name.
3. Kebab Case - Kebab case is similar to snake case, but you use a hyphen (-) instead of an underscore (_) to separate the words.
examples of kebab case: first-name and last-name.
4. Pascal Case - names in pascal case start with a capital letter. In case of the names with multiple words, all words will start with capital letters.
examples of pascal case: FirstName and LastName.
Variable:
· A variable is a container which holds the value while the Java program is executed.
· A variable is assigned with a data type.
· Variable is a name of memory location.
We can initialize using 3 components:
1)datatype: Type of data that can be stored in this variable
2)Variable_name: Name given to the variable
3)Value: It is the initial value stored in the variable
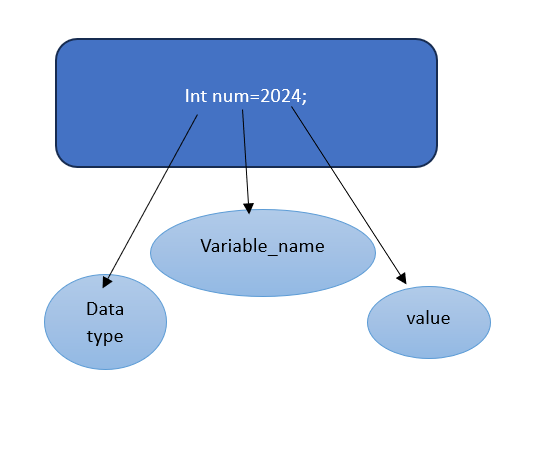
Types of Variable:
1.Local Variable
2.Instance Variables
3.Static Variables
Local Variables
A variable defined within a block or method or constructor is called a local variable.
The Local variable is created at the time of declaration and destroyed after exiting from the block or when the call returns from the function.
The scope of these variables exists only within the block in which the variables are declared, i.e., we can access these variables only within that block.
Initialization of the local variable is mandatory before using it in the defined scope
Instance Variables
Instance variables are non-static variables and are declared in a class outside of any method, constructor, or block.
As instance variables are declared in a class, these variables are created when an object of the class is created and destroyed when the object is destroyed.
Unlike local variables, we may use access specifiers for instance variables. If we do not specify any access specifier, then the default access specifier will be used.
Initialization of an instance variable is not mandatory. Its default value is dependent on the data type of variable. For String it is null, for float it is 0.0f, for int it is 0, for Wrapper classes like Integer it is null, etc.
Instance variables can be accessed only by creating objects.
Static Variables
Static variables are also known as class variables.
These variables are declared similarly to instance variables. The difference is that static variables are declared using the static keyword within a class outside of any method, constructor, or block.
Unlike instance variables, we can only have one copy of a static variable per class, irrespective of how many objects we create.
Static variables are created at the start of program execution and destroyed automatically when execution ends.
Initialization of a static variable is not mandatory.
If we access a static variable like an instance variable (through an object), the compiler will show a warning message, which won’t halt the program. The compiler will replace the object name with the class name automatically. The static variable is called only using class name.
If we access a static variable without the class name, the compiler will automatically append the class name. But for accessing the static variable of a different class, we must mention the class name as 2 different classes might have a static variable with the same name.
Static variables cannot be declared locally inside an instance method.
Static blocks can be used to initialize static variables.
Data types in Java
Computer memory values are measured in bit and bytes
Bit Vs Byte
A bit is the smallest unit of digital information, representing a binary value of either 0 or 1. On the other hand, a byte is a collection of 8 bits. It is commonly used to represent a single character or a small amount of data.
Data types in Java are of different sizes and values that can be stored in the variable that is made as per convenience and circumstances to cover up all test cases.
Java has two categories in which data types are segregated
1. Primitive Data Type: such as Boolean, char, int, short, byte, long, float, and double
2. Non-Primitive Data Type or Object Data type: such as String, Array, etc.
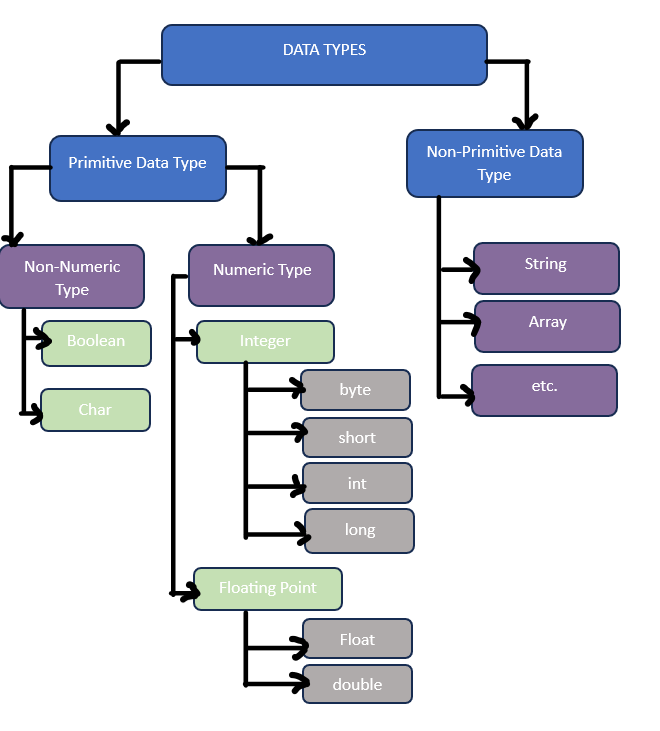
Primitive Data Types in Java
Primitive data are only single values and have no special capabilities. There are 8 primitive data types. (Size is fixed permanently across all)
Type | Description | Default | Range of values |
boolean | true or false | false | true,false |
byte | twos-complement integer | none | -128 to 127 |
char | ASCII values | '\u0000' | ASCII values 0 to 255 |
short | twos-complement integer | 0 | -32,768 to 32,767 |
int | twos-complement integer | 0 | -2,147,483,648 to 2,147,483,647 |
long | twos-complement integer | 0L | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | IEEE 754 floating point | 0.0f | upto 7 decimal digits |
double | IEEE 754 floating point | 0.0d | upto 16 decimal digits |
Non-Primitive Data Type or Reference Data Types
The Reference Data Types will contain a memory address of variable values because the reference types won’t store the variable value directly in memory. They are strings, objects, arrays, etc.
The main differences between primitive and non-primitive data types are:
Primitive types in Java are predefined and built into the language, while non-primitive types are created by the programmer (except for String).
Non-primitive types can be used to call methods to perform certain operations, whereas primitive types cannot.
Primitive types start with a lowercase letter (like int), while non-primitive types typically starts with an uppercase letter (like String).
Primitive types always hold a value, whereas non-primitive types can be null.
Examples of non-primitive types are Strings, Arrays, Classes etc.