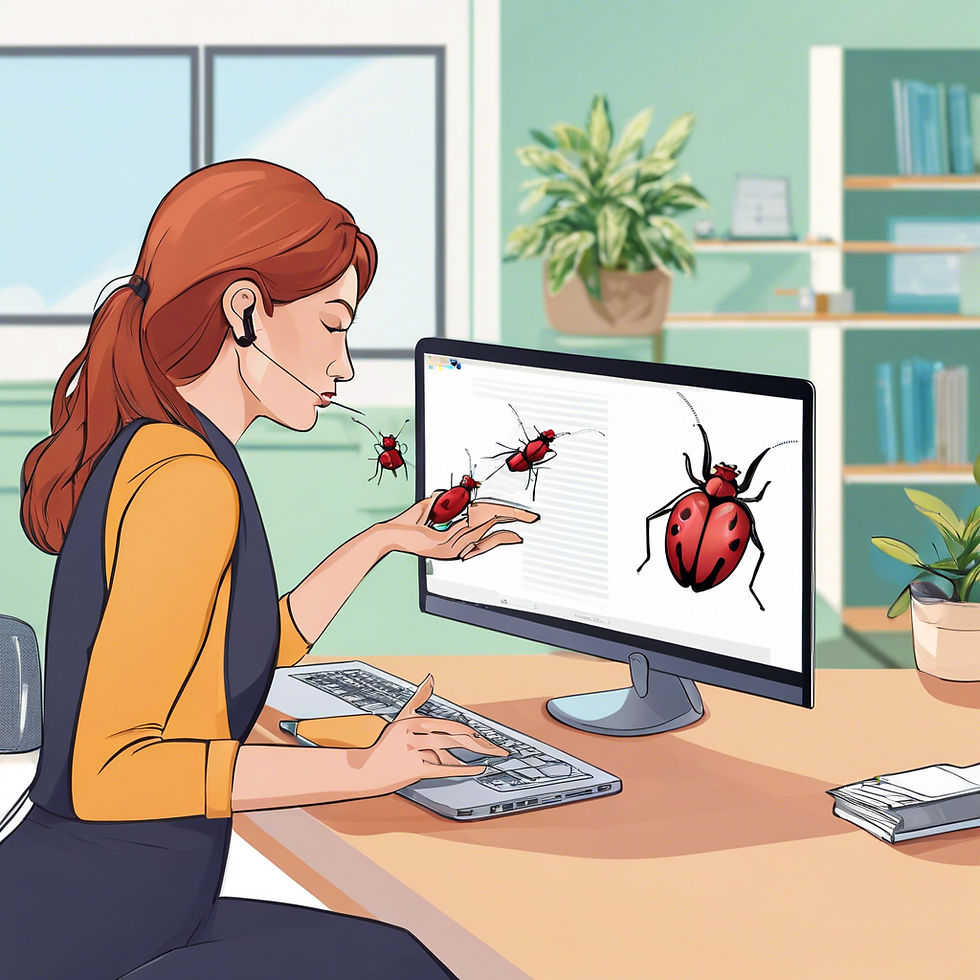
Debugging in everyday life
People debug and troubleshoot all the time. For example, when their babies cry, they check whether baby is hungry or diaper need a change.
Likewise Debugging is the process of finding and fixing errors or bugs in the source code of any software. When software does not work as expected, computer programmers study/debug the code to determine why any errors occurred.
The term "debugging" is linked to Admiral Grace Hopper, who in the 1940s referred to fixing a Mark II computer malfunction caused by a moth as "debugging." She documented it as the "First actual bug being found."
Debugging is different from testing. Testing focuses on finding bugs, errors, etc whereas debugging starts after a bug has been identified in the software.
Debug Setup: To start debugging a java program first we need to set a breakpoint, then activate Debug mode and then we start debugging using function keys to control the flow of execution.
Step 1: Start with setting Breakpoints
Know Breakpoints:
A breakpoint is a signal that tells the debugger to temporarily suspend execution of your program at a certain point in the code.
There are different ways you can put the breakpoint, but choosing one is very important for debugging.
Toggle Breakpoint: Setting and removing a breakpoint is called a toggling breakpoint.
Eclipse
Double-click on the left-hand side where all the number lines are to create a breakpoint. or place the caret at the line and press Ctrl+Shift+B or right click the gutter at the line of code and select 'Toggle Breakpoint'.
IntelliJ IDEA
Click the gutter at the line of code where you want to set the breakpoint or place the caret at the line and press Ctrl+F8.
Step 2: Activate Debug Mode
To activate debug mode, one has to run the application/program in debug mode.
Eclipse
Right click on the class having main method and select Debug As -> Java Application or press Alt + Shift + D, J.
While activating debug mode Eclipse will ask to switch to debug perspective, Press switch to confirm.
IntelliJ IDEA
Right click on the class having main method and select Debug or Press Shift + F9.
Step 3: Control the flow of execution using function keys or buttons in toolbar.
Eclipse IDE Debug buttons in toolbar of debug mode perspective:
IntelliJ debug buttons in toolbar:
Let's try debugging a simple java program with all information provided.
Here is simple java code with it's output:
package com.numpyninja.calculate; public class Calculator { public static void main(String[] args) { calculate(4, 2); } private static void calculate(int num1, int num2) { System.out.println("4 + 2 is: " + addTwoNum(num1, num2)); System.out.println("4 - 2 is: " + subtractFromNum(num2, num1)); System.out.println("4 X 2 is: " + multiplyTowNum(num1, num2)); System.out.println("4 / 2 is: " + divideFromNum(num1, num2)); } private static int addTwoNum(int num1, int num2) { return num1 + num2; } private static int subtractFromNum(int num1, int num2) { return num1 - num2; } private static int multiplyTowNum(int num1, int num2) { return num1 * num2; } private static int divideFromNum(int num1, int num2) { try { return num1/num2; } catch (Exception e) { System.out.println("something went wrong"); } return 0; } }
Output:
4 + 2 is: 6
4 - 2 is: -2
4 X 2 is: 8
4 / 2 is: 2
As you can see the addition, multiplication and division is working as expected in the output. but there is something wrong with the subtraction code.
Let's debug in IDE first in Eclipse and later in IntelliJ and see what's wrong and fix it.
Note: Go to 'Debug in IntelliJ' if not using Eclipse.
Step 1: Set debug point: Right click on left gutter of line and choose toggle Breakpoint to set a debug point.
Step 2: Run in debug mode and open debug perspective as: Notice I have setup the debug points at line number 6, 11 and 21 and choose debug As -> Java Application after right clicking the program having main method.
It will look like this once debug perspective opens on confirming to switch the perspective.
Step 3: Control the flow of Execution - using function keys
The execution of program will start from the first debug point in our case line 6 as shown in screen shot above.
Press F8 (Resume) to jump to line 11 directly, where we have another break point.
One can also press F6 or F5 to reach line 11 if we want to go line by line.
Notice in screen shot above the value in Variable window in the right-hand side is 4 for num1 and 2 for num2.
Press F5 (Step into) or F8(Resume) to reach line number 21 in eclipse.
Notice the control of flow reaches the line number 21 of program and the value of num1 is 2 and num2 is 4 in the variable window.
To inspect the result of 'num1 - num2 ' here, select 'num1 - num2' at line 21 and press Ctrl + Shift + I or right click and select 'Inspect' from different options provided on right clicking.
The result should be 2 instead of -2. Clearly the value passed to num1 and num2 is wrong. As at line 11 num1 value was 4 and num2 value was 2 but as the flow reaches line number 21, the value gets changed as num1 value to 2 and num2 value to 4.
Press F8(Resume) to resume the program or press stop to terminate it or press F6 (Step Over, to go line by line till end of program).
Fix the code and rerun using all 3 steps as mentioned above:
Let's fix line number 11 as show below by correcting the order of parameter passed from (num2, num1) to (num1, num2) and rerun the program in debug mode again.
After rerunning the code and performing the same steps as mentioned above, notice the inspection window shows the correct result as:
once finished on pressing F8, the final output of program is as:
4 + 2 is: 6
4 - 2 is: 2
4 X 2 is: 8
4 / 2 is: 2
Note: Make sure to run one debug at a moment and finish it before starting a new one.
You can skip below section if not using IntelliJ.
Debug in IntelliJ:
Step 1: Set a debug point:
Click on the line number 6, 11 and 21 left gutter to place the debug points.
Step 2: Activate debug mode: Right click and choose Debug 'Calculator.main()'
Step 3: Control the flow of execution
The execution of program will start from the first debug point in our case line 6:
Press F9 (Resume, to jump to next break point) which is line 11.
One can choose to press F8 or F7 to reach line 11 line by line.
IntelliJ shows the value of num1 as 4 and num2 as 2 at line 11.
Press F7 to go in the subtract method. (Since we have another break point at line 21, one can choose to press F9 to directly jump to line 21)
The control reaches line 21 as shown below:
Notice the value of num1 as 2 and num2 as 4 here.
This is causing the wrong result. To inspect it. press Alt + F8 or right click and select Evaluate Expression.
A new window will open as shown below. Type the expression we want to evaluate, in our case 'num1 -num2' and see result as -2.
Close evaluate window and Press F9 to finish the program.
See console for the output, which is:
4 + 2 is: 6
4 - 2 is: -2
4 X 2 is: 8
4 / 2 is: 2
To fix the code at line number 11 we need to pass the parameter in correct order.
subtractFromNum(num2, num1) -> subtractFromNum(num1, num2)
Rerun the program in debug mode again and see the evaluation of expression now.
We see we get correct output or evaluation as shown above.
Press F9 to finish the program and see output in console:
4 + 2 is: 6
4 - 2 is: 2
4 X 2 is: 8
4 / 2 is: 2
Summary and Next Steps
Debugging is an essential skill in software development, helping to identify and fix errors in code. We've covered the very basics of debugging.
Practical steps for debugging in Eclipse and IntelliJ IDEA include setting breakpoints, activating debug mode, and using function keys to control the execution flow of your program. Understanding and utilizing these features will enhance your ability to debug efficiently.
To improve your debugging skills, practice regularly in your preferred development environment. Familiarize yourself with the debugging tools and shortcuts available in Eclipse and IntelliJ IDEA.
For more detailed instructions and advanced techniques, refer to resources like the Eclipse Debugging Tutorial and IntelliJ Debugging Tutorial.
Happy debugging!