In programming world an exception is a problem that occurs during the execution of a program. If it is not handled properly then it may disrupt the normal execution of a program.
In real world there are situations that we don’t anticipate. For example, you get in your car and car won’t start or you are ready to go to the work and you won’t find your cell phone. A human can cope with such unforeseen circumstances easily. This is not the case with the code. A program must know how to handle these situations.
Real world exception examples
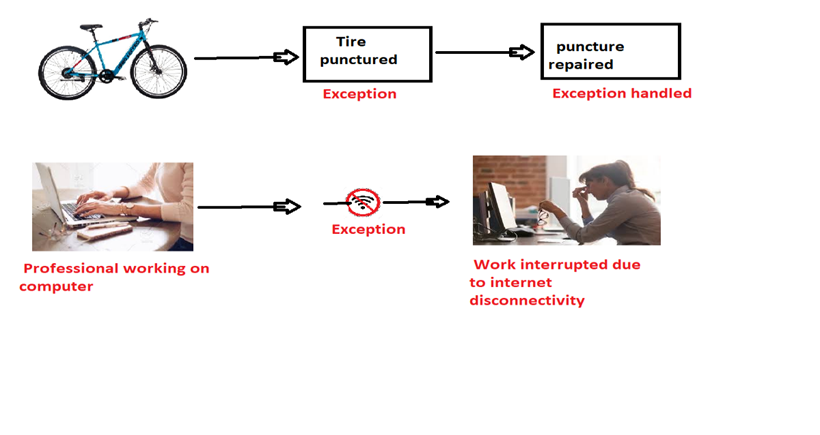
There are 2 types of exceptions in java:
Checked Exceptions: These exceptions occur at the compilation time. If we don’t handle checked exceptions, then it causes a compilation error, and the program will not compile.
Examples of most common checked exceptions:
ClassNotFoundException
IOException
SQLException
NoSuchMethodException
NoSuchElementException
InterruptedException
Unchecked Exceptions: These exceptions arise during run time. The program will compile but not execute.
Examples of most common unchecked exceptions:
ArrayIndexOutOfBoundException
ArithmeticException
NullPointerException
TypeNotPresentException
Difference between an error and exception

Java has a simple mechanism to handle these exceptions.
1. try..catch..finally block:
Syntax:
try {
// Code
} catch (Exception e) {
// code for exception
} finally {
// block always executes
}
Try block contains code that might generate an exception. Every try block is followed by a catch block.
An exception is caught by the catch block. Catch block cannot be used without the try block.
Any code that must be executed irrespective of occurrence of an exception is put in the finally block.
The finally block is always executed.
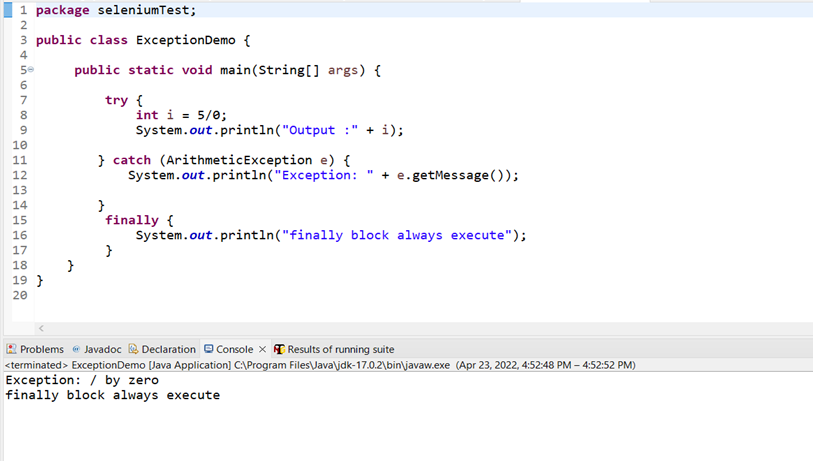
In the above code snippet, try block will throw an arithmetic exception which will be captured by the catch block. No matter what, finally block always execute as you can see the output in the console.
2. throws keyword:
For any method that can throw an exception, it is mandatory to use the “throws” keyword to list the exceptions that can be thrown. Throws keyword is always used after the method. Throws keyword will not handle the exception but it will suppress the exception.
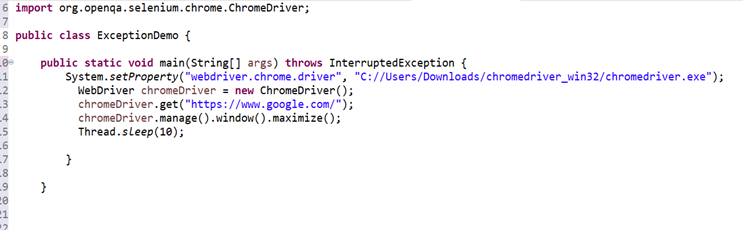
In the above code snippet, an interrupted exception is thrown when a thread is waiting, sleeping, or occupied to perform the normal execution of the code.
Conclusion:
Whenever you think some piece of code might throw an exception due to any reason, handle it with proper exception handling method so that normal execution of the program is not interrupted.