Object-oriented programming is an approach that provides a way of modularizing programs by creating partitioned memory area for both data and functions that can be used as templates for creating copies of such modules on demand.
OOP treats data as a critical element in the program development and does not allow it to flow freely around the system.
Objects and Classes:
An object is an instance of a class. It represents a real-world entity or concept and is created based on the blueprint defined by the class. Objects have their own unique set of attributes (fields) and can perform actions (invoke methods) defined by the class.
A class is a collection of objects of similar type. For example, mangoes, apple and orange are members of class fruit. Classes are user-defined data types and behave like the built-in types of a programming language.
Data Abstraction And Encapsulation:
The wrapping up of data and methods into a single unit (called class) is known as encapsulation.Data encapsulation is the most striking feature of a class.The data is not accessible to the outside word and only those methods,which are wrapped in the class,can access it.These methods provide the interface between the object’s data and the program.This insulation of the data from direct access by program is called data hiding.Encapsulation makes it possible for objects to be treated like ‘black boxes’,each performing a specific task without any concern for internal implementation.
Abstraction refers to the act of representing essential features without including the background details of explanations.Classes uses concept of abstraction and are defined as a list of abstract attributes such as size,weight and cost and methods that operate on these attributes.They encapsulate all the essential properties of the objects that are to be created.
Inheritance:
Java, Inheritance is an important pillar of OOP(Object-Oriented Programming). It is the mechanism in Java by which one class is allowed to inherit the features(fields and methods) of another class. In Java, Inheritance means creating new classes based on existing ones. A class that inherits from another class can reuse the methods and fields of that class. In addition, you can add new fields and methods to your current class as well.
Why Do We Need Java Inheritance?
· Code Reusability: The code written in the Superclass is common to all subclasses. Child classes can directly use the parent class code.
· Method Overriding: Method overriding is achievable only through Inheritance. It is one of the ways by which Java achieves Run Time Polymorphism.
· Abstraction: The concept of abstract where we do not have to provide all details is achieved through inheritance. Abstraction only shows the functionality to the user.
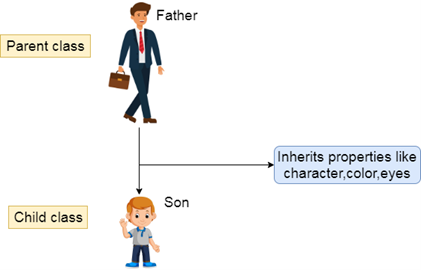
Property inheritance
Polymorphism:
The process of representing one form in multiple forms is known as Polymorphism.
Polymorphism is derived from 2 greek words: poly and morphs. The word "poly" means many and "morphs" means forms. So polymorphism means many forms.
Polymorphism is not a programming concept but it is one of the principal of OOPs. For many objects oriented programming language polymorphism principle is common but whose implementations are varying from one objects oriented programming language to another object oriented programming language.
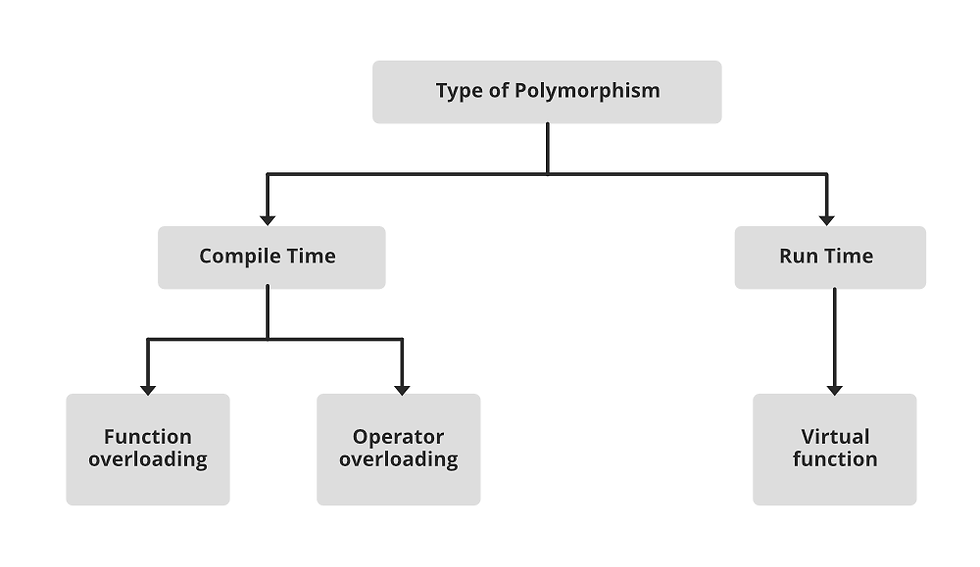
Real life example of polymorphism in Java:
You can see in the below Images, Man is only one, but he takes multiple roles like - he is a dad to his child, he is an employee, a salesperson and many more. This is known as Polymorphism.

How to achieve Polymorphism in Java ?
In java programming the Polymorphism principal is implemented with method overriding concept of java.
Polymorphism principal is divided into two sub principal they are:
· Static or Compile time polymorphism
· Dynamic or Runtime polymorphism
Static polymorphism in Java is achieved by method overloading and Dynamic polymorphism in Java is achieved by method overriding.
Benefits Of OOP:
OOP offers several benefits to both the program designer and the user. The Principal advantages are:
Through inheritance, we can eliminate redundant code and extend the use of existing classes.
The principle of data hiding helps the programmer to build secure program that cannot be invaded by code in other parts of the program.
It is possible to have multiple objects to coexist without any interference.
It is easy to partition the work in a project based on objects.
Software complexity can be easily managed.
It is possible to map objects in the problem domain to those objects in the program.
Message passing technique for communication between objects make the interface descriptions with external systems much simpler.